基于esp32的门禁系统
时间: 2024-08-13 22:06:16 浏览: 153
基于ESP32的门禁系统通常是一个物联网(IoT)应用,使用ESP32作为主控制器,结合RFID、磁卡读卡器或者其他身份验证设备,用于控制出入口的访问权限。以下是构建这样一个系统的简要步骤:
1. **硬件组成**:
- ESP32开发板: 作为核心处理和无线通信模块。
- RFID读卡模块或磁卡读卡器: 用户识别装置,读取用户权限信息。
- 门锁控制模块: 可能包括继电器或电机驱动器,用于锁定和解锁门。
- 电源、传感器和蜂鸣器等辅助组件。
2. **软件开发**:
- 使用MicroPython或Arduino IDE编写代码,管理数据交互和用户认证。
- 设计用户数据库,存储授权的卡片ID或RFID标识。
- 当用户刷卡或输入密码时,验证其身份。
- 根据验证结果,控制门锁开关。
- 可能还包括实时监控和报警功能。
3. **网络连接**:
- 如果需要远程监控,可以连接WiFi或蓝牙,通过云端平台实时查看门禁状态。
4. **安全措施**:
- 数据加密传输,保护用户的隐私。
- 实现防暴力破解机制,如多次尝试失败后锁定一段时间。
相关问题
基于ESP8266门禁系统显示器代码
以下是基于ESP8266门禁系统显示器的代码示例:
```
#include <ESP8266WiFi.h>
#include <WiFiClient.h>
#include <ESP8266WebServer.h>
#include <ESP8266HTTPClient.h>
#include <LiquidCrystal_I2C.h>
// Replace with your network credentials
const char* ssid = "Your_SSID";
const char* password = "Your_PASSWORD";
// Set web server port number to 80
ESP8266WebServer server(80);
// Set LCD pins
LiquidCrystal_I2C lcd(0x3F, 16, 2); // Set the LCD I2C address and dimensions
String serverUrl = "http://your-server-url.com/"; // Replace with your server URL
void setup() {
// Initialize Serial Monitor
Serial.begin(115200);
// Connect to Wi-Fi
WiFi.begin(ssid, password);
while (WiFi.status() != WL_CONNECTED) {
delay(1000);
Serial.println("Connecting to WiFi...");
}
Serial.println("Connected to WiFi");
// Start LCD
lcd.init(); // Initialize the LCD
lcd.backlight(); // Turn on the backlight
lcd.setCursor(0, 0); // Set the cursor to the first row
lcd.print("Connected to WiFi"); // Display message on LCD
}
void loop() {
// Handle client requests
server.handleClient();
// Check if there is a new message from the server
HTTPClient http;
http.begin(serverUrl); // Specify the URL
int httpCode = http.GET(); // Make the request
if (httpCode == 200) { // Check if the response is successful
String message = http.getString(); // Get the message
Serial.println(message); // Print the message to Serial Monitor
lcd.clear(); // Clear the LCD
lcd.setCursor(0, 0); // Set the cursor to the first row
lcd.print(message); // Display the message on the LCD
}
http.end(); // Close the connection
}
```
这个示例代码连接到Wi-Fi网络,然后初始化LCD显示器。它会从服务器获取消息并在LCD上显示。你需要把`Your_SSID`和`Your_PASSWORD`替换成你的Wi-Fi网络凭据,把`your-server-url.com`替换成你的服务器URL。
基于ESP8266门禁系统显示器读卡部分代码
以下是一个基于ESP8266门禁系统显示器读卡部分的代码示例:
```c++
#include <ESP8266WiFi.h>
#include <Wire.h>
#include <Adafruit_SSD1306.h>
#include <MFRC522.h>
#define OLED_RESET 0
Adafruit_SSD1306 display(OLED_RESET); //创建SSD1306 OLED显示器实例
#define SS_PIN 4
#define RST_PIN 5
MFRC522 mfrc522(SS_PIN, RST_PIN); //创建MFRC522实例
const char* ssid = "WiFi名称";
const char* password = "WiFi密码";
void setup() {
Serial.begin(9600); //初始化串口通信
display.begin(SSD1306_SWITCHCAPVCC, 0x3C); //初始化OLED显示器
display.clearDisplay(); //清空显示器
display.setTextSize(2); //设置字体大小
display.setTextColor(WHITE); //设置字体颜色
display.setCursor(0, 0); //设置光标位置
display.println("门禁系统"); //显示开机信息
display.display(); //显示到OLED
WiFi.begin(ssid, password); //连接WiFi
while (WiFi.status() != WL_CONNECTED) { //等待连接成功
delay(1000);
}
Serial.println("WiFi连接成功!");
Serial.println(WiFi.localIP());
display.clearDisplay(); //清空显示器
display.setCursor(0, 0); //设置光标位置
display.println("WiFi连接成功!"); //显示连接成功信息
display.display(); //显示到OLED
SPI.begin(); //初始化SPI总线
mfrc522.PCD_Init(); //初始化MFRC522
}
void loop() {
if (mfrc522.PICC_IsNewCardPresent() && mfrc522.PICC_ReadCardSerial()) { //检测到新的RFID卡
Serial.print("RFID卡UID: ");
for (byte i = 0; i < mfrc522.uid.size; i++) { //打印UID
Serial.print(mfrc522.uid.uidByte[i] < 0x10 ? "0" : "");
Serial.print(mfrc522.uid.uidByte[i], HEX);
}
Serial.println();
display.clearDisplay(); //清空显示器
if (mfrc522.uid.uidByte[0] == 0x12 && mfrc522.uid.uidByte[1] == 0x34 && mfrc522.uid.uidByte[2] == 0x56 && mfrc522.uid.uidByte[3] == 0x78) { //示例卡的UID为0x12 0x34 0x56 0x78
display.setCursor(0, 0); //设置光标位置
display.println("欢迎光临!"); //显示欢迎信息
display.display(); //显示到OLED
delay(1000); //延时1秒
} else {
display.setCursor(0, 0); //设置光标位置
display.println("无权限!"); //显示无权限信息
display.display(); //显示到OLED
delay(1000); //延时1秒
}
}
mfrc522.PICC_HaltA(); //停止感应卡
mfrc522.PCD_StopCrypto1(); //关闭加密
}
```
这段代码使用了Adafruit_SSD1306库进行OLED显示器的控制,实现了在检测到RFID卡时在显示器上显示欢迎信息或无权限信息。需要注意的是,示例中使用了一个固定的UID来判断授权,实际应用中需要根据具体需求进行修改。同时,需要根据实际情况修改代码中的WiFi连接信息和引脚定义。
阅读全文
相关推荐
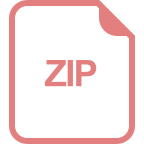
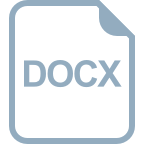
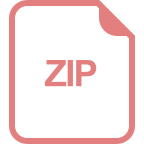
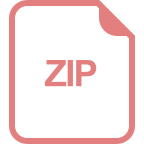
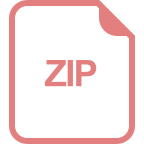
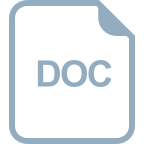
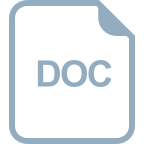
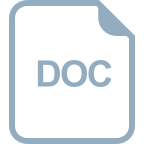
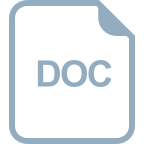
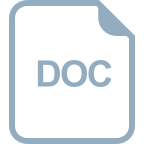
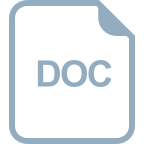
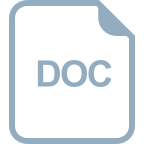

