在类组件中使用onCell
时间: 2024-10-20 17:15:09 浏览: 7
在React Native中,`onCell`通常用于表格组件(例如`FlatList`、`SectionList`等)中,它是一个事件处理器函数,当用户点击表格中的单元格时会被调用。当你需要对每个单元格的交互进行自定义处理时,可以在列配置对象中设置`onCell`属性。
例如,在`FlatList`中,你可以这样定义:
```jsx
<FlatList
data={yourData}
renderItem={({ item }) => (
<TouchableOpacity onPress={() => this.handleCellPress(item)}>
{/* 渲染单元格内容 */}
<Text>{item.title}</Text>
</TouchableOpacity>
)}
onCell={(item) => {
// 这里可以获取到点击的单元格数据,并触发更复杂的操作
console.log('Clicked cell:', item);
}}
/>
```
在这个例子中,每当用户点击单元格,`handleCellPress`函数会处理点击事件,而`onCell`则捕获并记录了点击的数据。
相关问题
我想在表格组件使用 oncell属性合并单元格,参数有record,index,请提供示例
好的,下面是一个示例代码,演示如何在表格组件中使用 oncell 属性合并单元格,具体实现方式如下:
```jsx
import { Table } from 'antd';
const dataSource = [
{
key: '1',
name: 'John Brown',
age: 32,
address: 'New York Park',
},
{
key: '2',
name: 'Jim Green',
age: 42,
address: 'London Park',
},
{
key: '3',
name: 'Joe Black',
age: 32,
address: 'Sidney Park',
},
{
key: '4',
name: 'Jim Red',
age: 32,
address: 'London Park',
},
];
const columns = [
{
title: 'Name',
dataIndex: 'name',
key: 'name',
render: (text, record, index) => {
const obj = {
children: text,
props: {},
};
if (index === 1) {
obj.props.rowSpan = 2;
}
// These two lines are used to merge cells vertically
if (index === 2 || index === 3) {
obj.props.rowSpan = 0;
}
return obj;
},
},
{
title: 'Age',
dataIndex: 'age',
key: 'age',
},
{
title: 'Address',
dataIndex: 'address',
key: 'address',
},
];
function App() {
return (
<Table
dataSource={dataSource}
columns={columns}
pagination={false}
onCell={(record, rowIndex) => {
return {
onClick: (event) => {}, // 点击行
onDoubleClick: (event) => {}, // 双击行
onContextMenu: (event) => {}, // 右键点击行
onMouseEnter: (event) => {}, // 鼠标移入行
onMouseLeave: (event) => {}, // 鼠标移出行
// 这里可以使用 record 和 rowIndex 来进行单元格合并操作
};
}}
/>
);
}
export default App;
```
在这个示例中,我们在表格的 `columns` 属性中,使用了 `render` 方法对 `Name` 列进行了单元格合并操作,实现了在第二行合并两个单元格的效果。
同时,我们在 `onCell` 属性中,使用了 `record` 和 `rowIndex` 两个参数,来实现单元格的合并操作,具体实现可以根据实际需求进行调整。
ant design onCell如何使用
Ant Design中Table组件的onCell属性可以通过以下方式使用:
1. 在Table组件标签中添加onCell属性:
```jsx
import { Table } from 'antd';
function App() {
const dataSource = [
{
key: '1',
name: 'John Brown',
age: 32,
address: 'New York',
},
{
key: '2',
name: 'Jim Green',
age: 42,
address: 'London',
},
];
const columns = [
{
title: 'Name',
dataIndex: 'name',
key: 'name',
onCell: (record, rowIndex) => {
return {
onClick: () => {
console.log(`clicked row: ${rowIndex}, name: ${record.name}`);
},
};
},
},
{
title: 'Age',
dataIndex: 'age',
key: 'age',
},
{
title: 'Address',
dataIndex: 'address',
key: 'address',
},
];
return <Table dataSource={dataSource} columns={columns} />;
}
```
在这个例子中,我们在第一列的onCell属性中定义了一个回调函数,当单击单元格时会触发该回调函数,并输出点击的行号和姓名。
2. 在columns中定义onCell属性:
```jsx
import { Table } from 'antd';
function App() {
const dataSource = [
{
key: '1',
name: 'John Brown',
age: 32,
address: 'New York',
},
{
key: '2',
name: 'Jim Green',
age: 42,
address: 'London',
},
];
const columns = [
{
title: 'Name',
dataIndex: 'name',
key: 'name',
},
{
title: 'Age',
dataIndex: 'age',
key: 'age',
},
{
title: 'Address',
dataIndex: 'address',
key: 'address',
onCell: (record, rowIndex) => {
return {
onClick: () => {
console.log(`clicked row: ${rowIndex}, address: ${record.address}`);
},
};
},
},
];
return <Table dataSource={dataSource} columns={columns} />;
}
```
在这个例子中,我们在第三列的onCell属性中定义了一个回调函数,当单击单元格时会触发该回调函数,并输出点击的行号和地址。
可以看到,无论是在Table组件标签中添加onCell属性,还是在columns中定义onCell属性,都可以实现对Table组件单元格的单击事件进行处理。
阅读全文
相关推荐
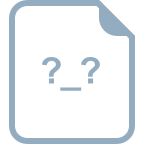
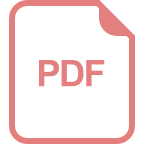
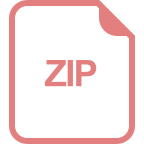
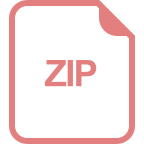
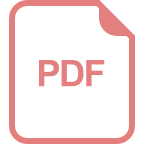
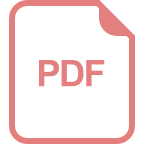
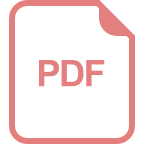
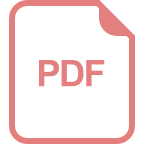
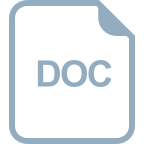
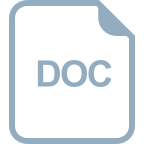
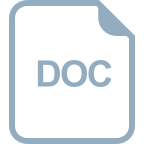





