以下是一个基于QgsMapTool和QgsRubberBand的自定义工具子类的示例代码,用于根据用户在屏幕上的点选位置顺序绘制双线条样式,并使用QgsRubberBand对象进行绘制。双线条的两条线以用户点选的线为中轴线,向两侧拓展10个像素。当选择完毕后,用户双击鼠标中间,将绘制的QgsRubberBand对象转化为QgsFeature对象并添加到图层QgsVectorLayer* mVectorLayer中。
时间: 2024-04-14 09:28:26 浏览: 16
以下是一个基于QgsMapTool和QgsRubberBand的自定义工具子类的示例代码,用于根据用户在屏幕上的点选位置顺序绘制双线条样式,并使用QgsRubberBand对象进行绘制。双线条的两条线以用户点选的线为中轴线,向两侧拓展10个像素。当选择完毕后,用户双击鼠标中间,将绘制的QgsRubberBand对象转化为QgsFeature对象并添加到图层QgsVectorLayer* mVectorLayer中。
```cpp
#include <QgsMapTool.h>
#include <QgsRubberBand.h>
#include <QMouseEvent>
#include <QPainter>
class CustomMapTool : public QgsMapTool
{
public:
CustomMapTool(QgsMapCanvas* canvas, QgsVectorLayer* vectorLayer)
: QgsMapTool(canvas), mVectorLayer(vectorLayer)
{
mRubberBand = new QgsRubberBand(canvas, QgsWkbTypes::LineString);
mRubberBand->setColor(Qt::red);
mRubberBand->setWidth(2);
}
void canvasPressEvent(QMouseEvent* event) override
{
if (event->button() == Qt::LeftButton)
{
// 添加点到RubberBand
QgsPointXY mapPoint = toMapCoordinates(event->pos());
mRubberBand->addPoint(mapPoint, false);
mPoints.append(mapPoint);
}
}
void canvasDoubleClickEvent(QMouseEvent* event) override
{
if (event->button() == Qt::LeftButton)
{
if (mPoints.count() > 1)
{
// 创建双线条的点集合
QgsRubberBand* doubleBand = new QgsRubberBand(mCanvas, QgsWkbTypes::LineString);
doubleBand->setColor(Qt::blue);
doubleBand->setWidth(2);
// 计算双线条的点坐标
QgsPointXY startPoint = mPoints.first();
QgsPointXY endPoint = mPoints.last();
double dx = endPoint.x() - startPoint.x();
double dy = endPoint.y() - startPoint.y();
double length = std::sqrt(dx * dx + dy * dy);
double dxNorm = dx / length;
double dyNorm = dy / length;
QgsPointXY offset(dxNorm * 10, dyNorm * 10);
// 添加左侧线条的点
for (const QgsPointXY& point : mPoints)
{
QgsPointXY leftPoint = point - offset;
doubleBand->addPoint(leftPoint, false);
}
// 添加右侧线条的点
for (const QgsPointXY& point : mPoints)
{
QgsPointXY rightPoint = point + offset;
doubleBand->addPoint(rightPoint, false);
}
// 结束RubberBand的编辑
doubleBand->endGeometry();
// 创建QgsFeature并添加到图层
QgsFeature feature;
feature.setGeometry(doubleBand->asGeometry());
mVectorLayer->dataProvider()->addFeatures({ feature });
// 清除临时绘制的RubberBand
mRubberBand->reset();
// 清除点集合
mPoints.clear();
}
}
}
void canvasMoveEvent(QMouseEvent* event) override
{
// 更新RubberBand的最后一个点
QgsPointXY mapPoint = toMapCoordinates(event->pos());
mRubberBand->movePoint(mapPoint);
}
void deactivate() override
{
// 重置RubberBand和点集合
mRubberBand->reset();
mPoints.clear();
}
private:
QgsVectorLayer* mVectorLayer;
QgsRubberBand* mRubberBand;
QList<QgsPointXY> mPoints;
};
```
您可以使用此示例代码作为起点,根据自己的需求进行修改和扩展。请确保在使用代码时,将QgsVectorLayer* mVectorLayer替换为您实际的矢量图层对象。
希望这对您有所帮助!如果您有任何进一步的问题,请随时提问。
相关推荐
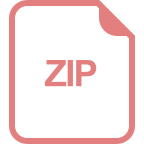
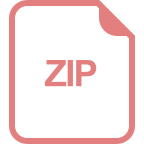
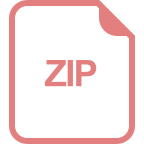














