pytorch RMSEloss
时间: 2023-10-18 13:29:47 浏览: 199
在PyTorch中,RMSELoss(均方根误差损失)是一种常用的回归损失函数。它用于衡量预测值与目标值之间的差异,并计算它们之间的均方根误差。
以下是一个使用PyTorch计算RMSELoss的示例:
```python
import torch
import torch.nn as nn
# 定义预测值和目标值(示例数据)
predictions = torch.tensor([0.9, 1.2, 2.1, 3.5], dtype=torch.float32)
targets = torch.tensor([1.0, 1.5, 2.0, 4.0], dtype=torch.float32)
# 计算均方根误差损失
criterion = nn.MSELoss() # 使用均方误差损失函数
rmse_loss = torch.sqrt(criterion(predictions, targets))
print(rmse_loss)
```
在上述代码中,我们首先定义了预测值(predictions)和目标值(targets)。然后,我们使用`nn.MSELoss`定义了一个均方误差损失函数。最后,通过使用`torch.sqrt`函数计算出均方根误差损失(RMSELoss)。
请注意,RMSELoss是基于MSELoss的计算结果而得出的。因此,在计算RMSELoss之前,我们需要先计算MSELoss,然后再取其平方根。
希望以上示例对您有所帮助!如果您有任何其他问题,请随时提问。
相关问题
pytorch rmseloss
PyTorch RMSELoss is a PyTorch loss function that calculates the root mean squared error between the predicted and the actual target values. It is useful for regression tasks, where the goal is to predict continuous values rather than discrete classes. The RMSELoss function is defined as the square root of the mean squared error, and it penalizes large errors more severely than small errors.
pytorch编写rmseloss
好的,以下是使用 PyTorch 编写 RMSLE Loss 的示例代码:
```python
import torch
import torch.nn as nn
# 定义 RMSLE Loss
class RMSLELoss(nn.Module):
def __init__(self):
super(RMSLELoss, self).__init__()
# 初始化 MSELoss
self.mse = nn.MSELoss()
def forward(self, predict, target):
# 计算 MSELoss
mse_loss = self.mse(torch.log1p(predict), torch.log1p(target))
# 计算 RMSLE Loss
rmsle_loss = torch.sqrt(mse_loss)
return rmsle_loss
```
在上述代码中,我们首先定义了 RMSLELoss 类,继承自 PyTorch 中的 nn.Module 类。在 RMSLELoss 类的构造函数中,我们初始化了 PyTorch 中的 MSELoss 类,因为 RMSLE Loss 是基于 MSELoss 计算得出的。
接着在 forward 方法中,我们首先使用 PyTorch 中的 log1p 函数对 predict 和 target 进行操作,然后调用之前初始化的 MSELoss 计算 MSELoss,并最终通过 PyTorch 中的 sqrt 函数计算出 RMSLE Loss。
这样,我们就成功地使用 PyTorch 编写了 RMSLE Loss。
阅读全文
相关推荐
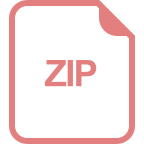
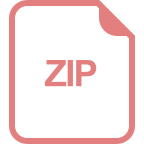
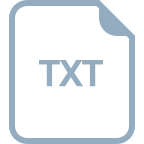
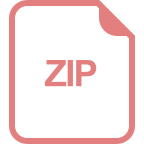
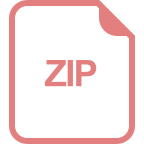
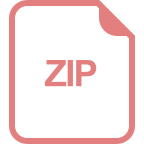
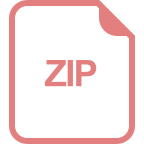
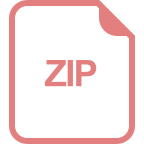
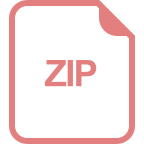
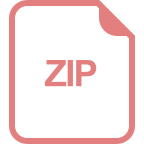
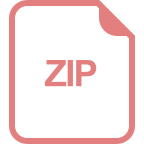