c语言怎么输入只含字母的字符串
时间: 2024-09-15 15:09:05 浏览: 56
在 C 语言中,你可以使用标准库函数 `scanf` 或者 `fgets` 来输入只包含字母的字符串。这里有两个示例:
1. 使用 `scanf` 函数:
```c
#include <stdio.h>
#include <ctype.h> // 引入 isalpha 函数
int main() {
char str[50]; // 定义一个足够大的字符数组来存储字符串
printf("请输入只含字母的字符串:");
while (scanf("%c", &str[0]) != EOF && isspace(str[0])) continue; // 跳过空白字符
if (!isalpha(str[0])) return 1; // 如果第一个字符不是字母则返回错误
for (int i = 1; scanf("%c", &str[i]) == 1 && isalpha(str[i]); i++) {} // 逐个读取并检查字符,直到遇到非字母字符或者换行符
str[i] = '\0'; // 结束字符串,添加空字符
printf("输入的字符串是:%s\n", str);
return 0;
}
```
在这个例子中,我们先跳过所有空白字符,然后检查每个输入的字符是否为字母。
2. 使用 `fgets` 函数:
```c
#include <stdio.h>
#include <ctype.h>
int main() {
char str[50];
printf("请输入只含字母的字符串:");
fgets(str, sizeof(str), stdin); // 从标准输入读取字符串,不包括换行符
int i = 0;
while (i < strlen(str) && !isspace(str[i]) && !isalpha(str[i])) { // 检查字符串中的每个字符
i++;
}
str[i] = '\0'; // 去掉结尾的换行符
printf("输入的字符串是:%s\n", str);
return 0;
}
```
这个版本也通过遍历字符串并判断字符性质来过滤非字母字符。
阅读全文
相关推荐


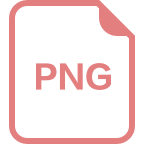













