如何用C++实现外币金额的汇总功能?
时间: 2024-11-09 16:30:17 浏览: 24
在C++中,实现外币金额的汇总功能通常涉及到货币转换和数据结构的使用。假设我们有一个包含不同货币的数组或容器,并且需要将它们转换成统一的货币(比如美元),我们可以按照以下步骤操作:
1. 定义一个结构体或类`CurrencyAmount`,包含货币类型(如枚举或字符串)和相应的金额(通常是double类型的数值)。
```cpp
struct CurrencyAmount {
std::string currency;
double amount;
};
```
2. 如果需要实时汇率,可以使用外部API(如Open Exchange Rates API)获取。这里你可以选择存储汇率到一个map或者vector中,键是货币代码,值是对应的汇率。
3. 对于每个外币金额,检查其是否已在汇率表中。如果存在,乘以其对应的汇率;如果没有,你需要添加一个新的汇率条目并计算。
4. 使用一个累加器(如`std::accumulate`函数)遍历所有转换后的美元金额求和。
5. 最后,返回总金额。
示例代码:
```cpp
#include <iostream>
#include <vector>
#include <map>
// 假设你已经有了一个获取汇率的函数getExchangeRate()
double getExchangeRate(const std::string& fromCurrency, const std::string& toCurrency);
std::vector<CurrencyAmount> currencies = {/*...*/};
double totalInUSD() {
std::map<std::string, double> exchangeRates; // 初始化汇率
double total = 0;
for (const auto& currency : currencies) {
if (exchangeRates.find(currency.currency) != exchangeRates.end()) {
total += currency.amount * exchangeRates[currency.currency];
} else {
exchangeRates[currency.currency] = getExchangeRate("USD", currency.currency);
total += currency.amount * exchangeRates[currency.currency];
}
}
return total;
}
int main() {
double result = totalInUSD();
std::cout << "Total in USD: " << result << std::endl;
return 0;
}
```
阅读全文
相关推荐
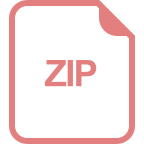
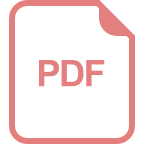
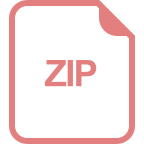
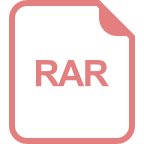
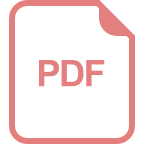
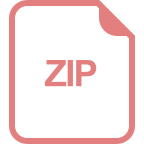
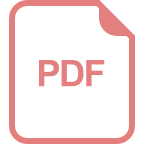
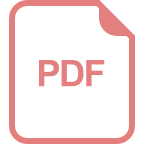
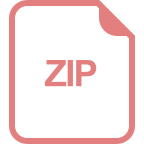
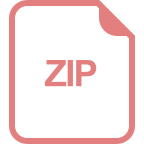
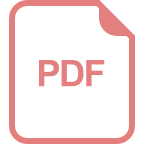
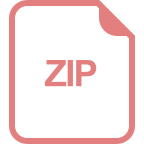
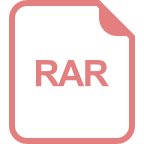