C++ string 获取字符串长度
时间: 2023-11-21 11:05:27 浏览: 102
可以使用C++中的`string`类来获取字符串长度,`string`类中提供了`length()`和`size()`两个函数来获取字符串长度,两个函数的用法是相同的,都可以返回字符串中字符的个数,包括空格在内。下面是一个例子:
```c++
#include <iostream>
#include <string>
using namespace std;
int main() {
string str = "Hello World!";
int len = str.length(); // 或者使用 str.size();
cout << "字符串长度为:" << len << endl;
return 0;
}
```
输出结果为:
```
字符串长度为:12
```
相关问题
devc++如何获取字符串长度
在 Dev-C++ 中,可以使用 `strlen` 函数来获取一个字符串的长度。`strlen` 函数位于 `string.h` 头文件中,可以通过在代码中添加 `#include <string.h>` 来引入该函数。
以下是一个示例代码,演示了如何使用 `strlen` 函数来获取字符串的长度:
```c
#include <stdio.h>
#include <string.h>
int main() {
char str[] = "Hello, world!";
int len = strlen(str);
printf("The length of the string is: %d\n", len);
return 0;
}
```
上述代码定义了一个字符数组 `str`,并使用 `strlen` 函数获取了字符串的长度,最后将长度输出到屏幕上。在 Dev-C++ 中编译并运行该代码,输出结果应为:
```
The length of the string is: 13
```
C++获取string字符串的长度
在C++中,有多种方法可以获取`std::string`类型的字符串长度。以下是三种常见的方式:
1. 使用`length()`或`size()`成员函数[^1]:
```cpp
#include <string>
#include <iostream>
using namespace std;
int main() {
string str = "my string";
cout << str.length() << endl; // 输出字符串长度
// 或者使用 size() 函数,效果相同
cout << str.size() << endl;
return 0;
}
```
2. 使用`strlen()`函数,但需要先通过`c_str()`转换成`const char*`:
```cpp
#include <string>
#include <iostream>
using namespace std;
int main() {
string str = "Test string";
const char* cstr = str.c_str();
cout << strlen(cstr) << endl; // 输出字符串长度(注意这不是直接从std::string得到的)
return 0;
}
```
阅读全文
相关推荐
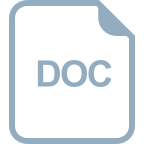
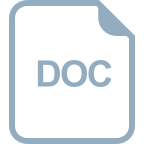
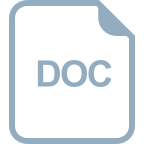













