C语言是先输入一个字符串然后按照字母升序排序后输出
时间: 2024-12-19 12:23:20 浏览: 6
C语言中对字符串进行字母升序排序通常需要借助数组或者动态内存管理。一种常见的做法是将字符串转换为字符数组,然后使用qsort()函数进行排序,最后再输出。
以下是一个简单的示例,假设我们有一个包含空格分隔单词的字符串:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
// 比较函数,用于qsort()
int compare(const void *a, const void *b) {
return (*(char **)a - *(char **)b);
}
void sort_string(char *str) {
// 将字符串按空格分割成单词
char *word = strtok(str, " ");
if (word == NULL) {
printf("No words in the string.\n");
return;
}
char **words = malloc(sizeof(char *) * 2); // 初始分配两个元素
words[0] = word;
while ((word = strtok(NULL, " ")) != NULL) {
words = realloc(words, sizeof(char *) * (strlen(words) + 2)); // 动态扩容
words[strlen(words)] = word;
}
// 排序单词
qsort(words, strlen(words), sizeof(char*), compare);
// 输出排序后的结果
for (int i = 0; words[i]; ++i) {
printf("%s ", words[i]);
}
free(words);
}
int main() {
char input[] = "hello world this is a test";
sort_string(input);
return 0;
}
```
在这个示例中,`compare()`函数实现了字典顺序比较,而`sort_string()`函数则负责处理整个过程。注意,这只是一个基本的示例,实际应用中可能需要处理更多边界情况和错误检查。
阅读全文
相关推荐
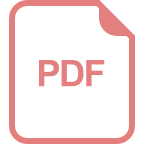
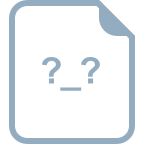
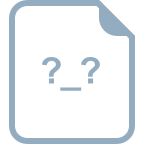















