实现一个圆柱体类,类中包含私有数据成员半径和高;包含设置圆柱体半径和高、计算圆柱体体积;获取圆柱体半径、高、圆柱体体积的成员函数;能够对数据有效性进行判断处理。编写主函数测试类的使用。
时间: 2024-12-22 22:12:25 浏览: 8
在C++中,你可以创建一个名为`Cylinder`的类来表示圆柱体,包含私有变量表示半径和高,以及公共方法用于设置属性、计算体积、获取属性并进行数据有效性检查。下面是一个简单的示例:
```cpp
#include <iostream>
#include <cmath> // 包含math库来进行数学运算
class Cylinder {
private:
double radius;
double height;
public:
// 构造函数,如果参数无效则默认值
Cylinder(double r = 0.0, double h = 0.0) :
radius(r > 0 ? r : 0), height(h > 0 ? h : 0) {}
// 设置圆柱体半径和高,检查是否有效
void setProperties(double newRadius, double newHeight) {
if (newRadius > 0 && newHeight > 0) {
radius = newRadius;
height = newHeight;
} else {
std::cerr << "Invalid values for radius or height!\n";
}
}
// 计算圆柱体体积
double calculateVolume() const {
return M_PI * pow(radius, 2) * height;
}
// 获取圆柱体半径
double getRadius() const {
return radius;
}
// 获取圆柱体高
double getHeight() const {
return height;
}
// 获取圆柱体体积
double getVolume() const {
return calculateVolume();
}
};
int main() {
// 创建一个圆柱体
Cylinder cylinder(3.5, 4.0);
// 设置新的属性
cylinder.setProperties(2.0, 5.0);
std::cout << "Radius: " << cylinder.getRadius() << ", Height: " << cylinder.getHeight()
<< ", Volume: " << cylinder.getVolume() << "\n";
// 使用无效值设置属性
cylinder.setProperties(-1.0, -1.0); // 将捕获错误
return 0;
}
```
在这个例子中,`Cylinder`类包含了半径和高的设置和获取方法,以及计算体积的方法。构造函数接受可选的初始值,并在构造时检查输入的有效性。在`main`函数中,我们创建了一个圆柱体实例,设置了正常和无效的属性,展示了如何使用类的方法。
阅读全文
相关推荐
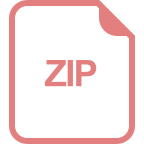
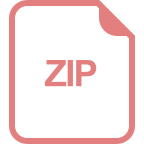
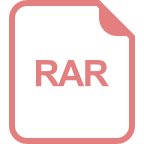















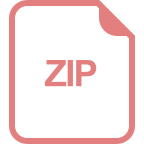