如何使用Python进行AES解密,要求把完整代码写出来
时间: 2024-10-16 20:06:40 浏览: 19
在Python中,可以使用`cryptography`库来进行AES(Advanced Encryption Standard)加密和解密操作。以下是一个简单的例子,展示了如何使用AES-CBC模式进行加密和解密:
```python
from cryptography.hazmat.primitives.ciphers import Cipher, algorithms, modes
from cryptography.hazmat.backends import default_backend
from cryptography.hazmat.primitives.kdf.pbkdf2 import PBKDF2HMAC
import base64
# 导入密钥和初始向量(IV)
key = b'some_random_key12345'
iv = b'some_initial_vector'
# 使用PBKDF2从密码生成密钥
salt = b'some_salt'
password = b'user_password'
kdf = PBKDF2HMAC(algorithm=algorithms.HMAC(algorithm=algorithms.SHA256(), length=32), salt=salt, iterations=100000, backend=default_backend())
derived_key = kdf.derive(password)
# 创建Cipher对象
cipher = Cipher(algorithms.AES(derived_key), modes.CBC(iv), backend=default_backend())
# 加密函数
def encrypt(text):
encryptor = cipher.encryptor()
ct_bytes = encryptor.update(text.encode()) + encryptor.finalize()
return base64.b64encode(iv + ct_bytes).decode()
# 解密函数
def decrypt(ciphertext):
decoded_ciphertext = base64.b64decode(ciphertext)
iv = decoded_ciphertext[:16]
ct_bytes = decoded_ciphertext[16:]
decryptor = cipher.decryptor()
pt_bytes = decryptor.update(ct_bytes) + decryptor.finalize()
return pt_bytes.decode('utf-8')
# 示例
plaintext = "Hello, world!"
encrypted_text = encrypt(plaintext)
print("Encrypted text:", encrypted_text)
decrypted_text = decrypt(encrypted_text)
print("Decrypted text:", decrypted_text)
阅读全文
相关推荐
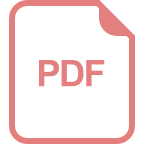
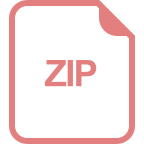
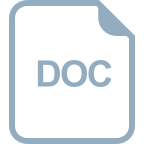














