java创建两个线程,要求如下: (1)一个线程输出100个1~26,另一个线程输出100个A~Z。 (2)一个线程使用集成Thread 类的写法,另一个线程使用实现Runnable接口的写法。 输出格式: 每一行输出一个1~26数字或者是A~Z的字符
时间: 2024-06-14 08:03:56 浏览: 151
以下是Java创建两个线程的示例代码:
1. 使用继承Thread类的写法:
```java
class NumberThread extends Thread {
@Override
public void run() {
for (int i = 1; i <= 100; i++) {
System.out.print(i + " ");
if (i % 26 == 0) {
System.out.println();
}
}
}
}
class LetterThread extends Thread {
@Override
public void run() {
for (char c = 'A'; c <= 'Z'; c++) {
System.out.print(c + " ");
if (c == 'Z') {
System.out.println();
}
}
}
}
public class Main {
public static void main(String[] args) {
NumberThread numberThread = new NumberThread();
LetterThread letterThread = new LetterThread();
numberThread.start();
letterThread.start();
}
}
```
2. 使用实现Runnable接口的写法:
```java
class NumberRunnable implements Runnable {
@Override
public void run() {
for (int i = 1; i <= 100; i++) {
System.out.print(i + " ");
if (i % 26 == 0) {
System.out.println();
}
}
}
}
class LetterRunnable implements Runnable {
@Override
public void run() {
for (char c = 'A'; c <= 'Z'; c++) {
System.out.print(c + " ");
if (c == 'Z') {
System.out.println();
}
}
}
}
public class Main {
public static void main(String[] args) {
Thread numberThread = new Thread(new NumberRunnable());
Thread letterThread = new Thread(new LetterRunnable());
numberThread.start();
letterThread.start();
}
}
```
阅读全文
相关推荐
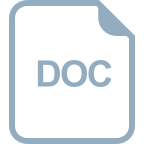














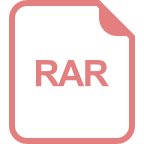

