如何用SQLite存储格式保存数据库文件
时间: 2024-04-16 17:25:02 浏览: 93
要使用SQLite存储格式保存数据库文件,你可以按照以下步骤进行操作:
1. 在你的C/C++项目中引入SQLite库。你可以从SQLite官方网站(https://www.sqlite.org/download.html)下载SQLite的预编译二进制文件,并将其添加到你的项目中。
2. 使用SQLite API打开数据库连接,并创建表格以存储数据。
```cpp
#include <sqlite3.h>
sqlite3* db;
int rc = sqlite3_open("path/to/database.db", &db);
if (rc != SQLITE_OK) {
printf("Error opening database: %s\n", sqlite3_errmsg(db));
return;
}
// 创建表格
const char* createTableQuery = "CREATE TABLE IF NOT EXISTS your_table (field1 TEXT, field2 INTEGER, ...)";
rc = sqlite3_exec(db, createTableQuery, 0, 0, 0);
if (rc != SQLITE_OK) {
printf("Error creating table: %s\n", sqlite3_errmsg(db));
sqlite3_close(db);
return;
}
```
3. 执行插入语句,将数据保存到数据库中。
```cpp
// 执行插入语句
const char* insertQuery = "INSERT INTO your_table (field1, field2, ...) VALUES (?, ?, ...)";
sqlite3_stmt* stmt;
rc = sqlite3_prepare_v2(db, insertQuery, -1, &stmt, 0);
if (rc != SQLITE_OK) {
printf("Error preparing statement: %s\n", sqlite3_errmsg(db));
sqlite3_close(db);
return;
}
while (query.next()) {
// 绑定参数
sqlite3_bind_text(stmt, 1, query.value(0).toString().toUtf8().constData(), -1, SQLITE_STATIC);
sqlite3_bind_int(stmt, 2, query.value(1).toInt());
// 绑定其他参数...
// 执行插入操作
rc = sqlite3_step(stmt);
if (rc != SQLITE_DONE) {
printf("Error inserting record: %s\n", sqlite3_errmsg(db));
sqlite3_finalize(stmt);
sqlite3_close(db);
return;
}
// 重置语句
sqlite3_reset(stmt);
}
sqlite3_finalize(stmt);
```
4. 关闭数据库连接。
```cpp
sqlite3_close(db);
```
请注意,以上代码只是示例,你需要根据实际情况进行适当的修改和调整。另外,SQLite提供了更多的API函数用于执行查询、更新和删除操作,你可以根据自己的需求进行使用。同时,你也可以使用SQLite提供的命令行工具或其他SQLite客户端工具来管理和查看保存在SQLite数据库中的数据。
阅读全文
相关推荐
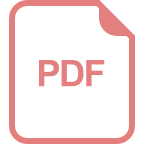
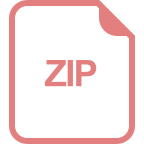
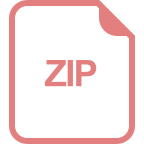
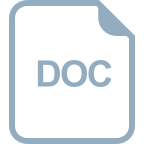
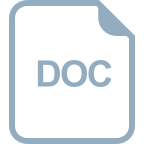
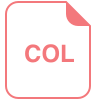
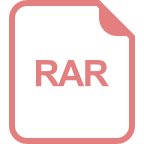
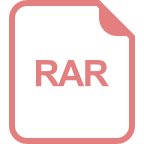
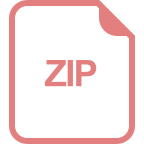
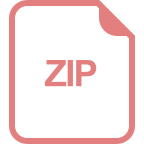
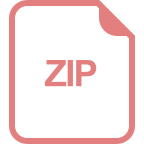
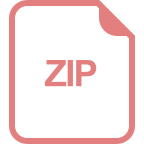
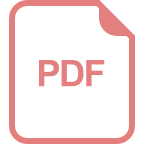
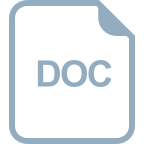
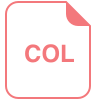
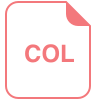