获取实现某接口方法的所有
时间: 2024-11-25 18:12:02 浏览: 11
在Java中,获取某个接口的所有实现方法并不是直接可用的,因为语言设计并不提供这样的内置功能。但是,你可以通过反射机制来动态地获取接口的所有实现类以及它们各自实现的方法。下面是一个简单的示例,假设我们有一个名为`MyInterface`的接口:
```java
import java.lang.reflect.Method;
interface MyInterface {
void method1();
void method2();
}
// 实现该接口的一些类
class Impl1 implements MyInterface {
@Override
public void method1() {
System.out.println("Impl1 - method1");
}
@Override
public void method2() {
System.out.println("Impl1 - method2");
}
}
class Impl2 implements MyInterface {
@Override
public void method1() {
System.out.println("Impl2 - method1");
}
@Override
public void method2() {
System.out.println("Impl2 - method2");
}
}
public class Main {
public static void main(String[] args) throws NoSuchMethodException {
Class<?> myInterfaceClass = MyInterface.class;
// 获取接口的所有直接实现类
for (Class<?> clazz : myInterfaceClass.getDeclaringClasses()) {
if (myInterfaceClass.isAssignableFrom(clazz)) {
System.out.println("Implementing class: " + clazz.getName());
// 获取所有公开的方法
Method[] methods = clazz.getDeclaredMethods();
for (Method method : methods) {
if (method.getAnnotation(MyInterface.class.getCanonicalName()) != null) {
System.out.println("\tMethod: " + method.getName());
}
}
}
}
}
}
```
运行上述代码会显示实现`MyInterface`的每个类及其对应的方法。然而,这种方法仅适用于接口的直接实现,如果存在间接实现或代理类,就不再有效了。
阅读全文
相关推荐
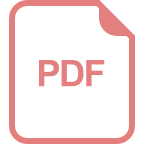
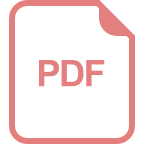
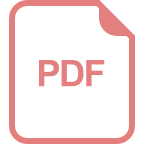
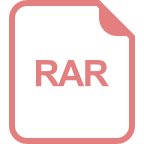
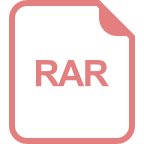
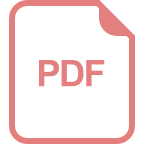
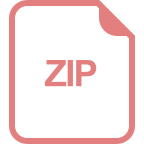
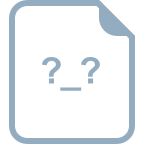
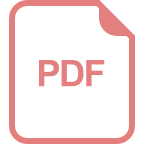
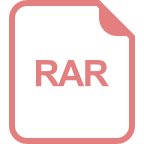
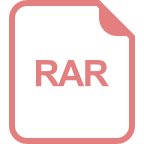
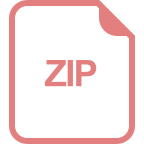
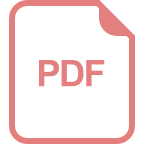
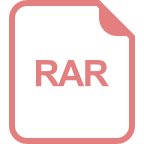
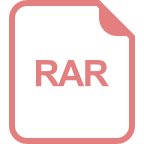
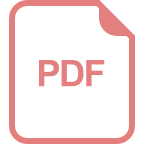
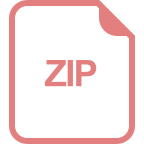