vue webview
时间: 2024-03-07 11:45:55 浏览: 17
Vue WebView是一个基于Vue.js框架的组件,用于在移动应用程序中嵌入Web视图。它允许你在Vue应用程序中加载和显示Web内容,类似于在浏览器中打开一个网页。
Vue WebView提供了一个容器,可以加载并显示Web页面。你可以使用它来展示网页、HTML5游戏、H5应用等。它可以与Vue.js的生命周期钩子函数结合使用,以便在加载和显示Web内容时执行相应的操作。
使用Vue WebView,你可以轻松地将Web内容嵌入到你的移动应用程序中,同时还可以利用Vue.js的强大功能来处理数据和逻辑。它提供了一种简单而灵活的方式来创建具有Web视图的移动应用程序。
相关问题
vue webview加载显示网页
要在Vue应用程序中加载和显示网页,可以使用Vue Webview组件。Vue Webview是一个基于Webview的Vue.js插件,可以将原生Webview嵌入Vue.js应用程序中。
首先,你需要安装Vue Webview插件。可以通过npm命令来安装:
```
npm install vue-webview
```
接下来,在你的Vue应用程序中导入Vue Webview组件:
```javascript
import VueWebview from 'vue-webview';
Vue.use(VueWebview);
```
然后,在Vue模板中使用Vue Webview组件来加载和显示网页:
```vue
<template>
<div>
<vue-webview :src="url"></vue-webview>
</div>
</template>
<script>
export default {
data() {
return {
url: 'https://www.example.com',
};
},
};
</script>
```
在上面的代码中,我们在Vue模板中使用了Vue Webview组件,并将网页的URL作为组件的属性传递给它。这将导致Webview加载并显示指定的网页。
注意:Webview组件只能在原生应用程序中使用,而不能在Web应用程序中使用。所以,如果你的Vue应用程序是一个Web应用程序,那么你需要将其打包为原生应用程序才能使用Webview组件。
vue webview跳转
Vue.js 中使用 webview 跳转可以通过以下步骤:
1. 在 Vue.js 的组件中添加一个 iframe,例如:
```html
<template>
<div>
<iframe ref="myWebView" :src="url"></iframe>
</div>
</template>
```
2. 在组件的 mounted 钩子函数中设置 iframe 的 src 属性为目标网址:
```javascript
mounted() {
this.$refs.myWebView.src = 'https://example.com';
}
```
3. 可以通过监听 iframe 的 load 事件来判断是否加载完成:
```javascript
mounted() {
this.$refs.myWebView.addEventListener('load', this.handleWebViewLoad);
},
methods: {
handleWebViewLoad() {
console.log('WebView loaded!');
}
}
```
注意:在使用 iframe 进行跨域跳转时,需要目标网站也支持跨域访问。否则,会出现跨域安全错误。
相关推荐
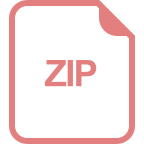
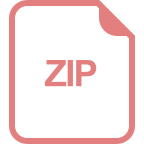












