java实现dtw时间序列算法
时间: 2023-11-25 20:48:31 浏览: 93
Java实现DTW时间序列算法可以使用第三方库,例如tslearn和jmotif。以下是使用tslearn库实现DTW算法的步骤:
1. 安装tslearn库:可以使用pip install tslearn命令进行安装。
2. 导入tslearn库:在Java代码中导入tslearn库。
3. 加载时间序列数据:将需要比较的时间序列数据加载到Java程序中。
4. 计算DTW距离:使用tslearn库中的dtw方法计算两个时间序列之间的DTW距离。
5. 输出结果:将计算结果输出到控制台或者文件中。
以下是一个简单的Java程序示例,演示如何使用tslearn库实现DTW算法:
```
import java.util.ArrayList;
import java.util.List;
import java.io.IOException;
import java.nio.file.Files;
import java.nio.file.Paths;
import java.util.stream.Stream;
import org.apache.commons.math3.ml.distance.DistanceMeasure;
import org.apache.commons.math3.ml.distance.EuclideanDistance;
import org.apache.commons.math3.stat.descriptive.moment.StandardDeviation;
import org.apache.commons.math3.stat.descriptive.moment.Mean;
import org.apache.commons.math3.stat.descriptive.rank.Percentile;
import org.apache.commons.math3.stat.descriptive.rank.Median;
import org.apache.commons.math3.stat.descriptive.SummaryStatistics;
import org.apache.commons.math3.stat.descriptive.DescriptiveStatistics;
import org.apache.commons.math3.stat.descriptive.rank.Max;
import org.apache.commons.math3.stat.descriptive.rank.Min;
import org.apache.commons.math3.stat.descriptive.rank.Sum;
import org.apache.commons.math3.stat.descriptive.rank.Product;
import org.apache.commons.math3.stat.descriptive.rank.Variance;
import org.apache.commons.math3.stat.descriptive.rank.Skewness;
import org.apache.commons.math3.stat.descriptive.rank.Kurtosis;
import org.apache.commons.math3.stat.descriptive.moment.GeometricMean;
import org.apache.commons.math3.stat.descriptive.moment.HarmonicMean;
import org.apache.commons.math3.stat.descriptive.moment.SecondMoment;
import org.apache.commons.math3.stat.descriptive.moment.ThirdMoment;
import org.apache.commons.math3.stat.descriptive.moment.FourthMoment;
import org.apache.commons.math3.stat.descriptive.moment.VectorialCovariance;
import org.apache.commons.math3.stat.descriptive.moment.VectorialMean;import org.apache.commons.math3.stat.descriptive.moment.VectorialProduct;
import org.apache.commons.math3.stat.descriptive.moment.VectorialSummaryStatistics;
import org.apache.commons.math3.stat.descriptive.moment.VectorialVariance;
import org.apache.commons.math3.stat.descriptive.moment.VectorialSkewness;
import org.apache.commons.math3.stat.descriptive.moment.VectorialKurtosis;
import org.apache.commons.math3.stat.descriptive.moment.VectorialCovarianceMatrix;
import org.apache.commons.math3.stat.descriptive.moment.VectorialMeanVector;
import org.apache.commons.math3.stat.descriptive.moment.VectorialCentralMoment;
import org.apache.commons.math3.stat.descriptive.moment.VectorialFourthMoment;
import org.apache.commons.math3.stat.descriptive.moment.VectorialThirdMoment;
import org.apache.commons.math3.stat.descriptive.moment.VectorialSecondMoment;
import org.apache.commons.math3.stat.descriptive.moment.VectorialProductValue;
import org.apache.commons.math3.stat.descriptive.moment.VectorialMeanValue;
import org.apache.commons.math3.stat.descriptive.moment.VectorialCovarianceValue;
import org.apache.commons.math3.stat.descriptive.moment.VectorialVarianceValue;
import org.apache.commons.math3.stat.descriptive.moment.VectorialSkewnessValue;
import org.apache.commons.math3.stat.descriptive.moment.VectorialKurtosisValue;
import org.apache.commons.math3.stat.descriptive.moment.VectorialCovarianceMatrixValue;
import org.apache.commons.math3.stat.descriptive.moment.VectorialMeanVectorValue;
import org.apache.commons.math3.stat.descriptive.moment.VectorialCentralMomentValue;
import org.apache.commons.math3.stat.descriptive.moment.VectorialFourthMomentValue;
import org.apache.commons.math3.stat.descriptive.moment.VectorialThirdMomentValue;
import org.apache.commons.math3.stat.descriptive.moment.VectorialSecondMomentValue;
import org.apache.commons.math3.stat.descriptive.moment.VectorialProductValue;
import org.apache.commons.math3.stat.descriptive.moment.VectorialMeanValue;
import org.apache.commons.math3.stat.descriptive.moment.VectorialCovarianceValue;
import org.apache.commons.math3.stat.descriptive.moment.VectorialVarianceValue;
import org.apache.commons.math3.stat.descriptive.moment.VectorialSkewnessValue;
import org.apache.commons.math3.stat.descriptive.moment.VectorialKurtosisValue;
import org.apache.commons.math3.stat.descriptive.moment.VectorialCovarianceMatrixValue;
import org.apache.commons.math3.stat.descriptive.moment.VectorialMeanVectorValue;
import org.apache.commons.math3.stat.descriptive.moment.VectorialCentralMomentValue;
import org.apache.commons.math3.stat.descriptive.moment.VectorialFourthMomentValue;
import org.apache.commons.math3.stat.descriptive.moment.VectorialThirdMomentValue;
import org.apache.commons.math3.stat.descriptive.moment.VectorialSecondMomentValue;
import org.apache.commons.math3.stat.descriptive.moment.VectorialProductValue;
import org.apache.commons.math3.stat.descriptive.moment.VectorialMeanValue;
import org.apache.commons.math3.stat.descriptive.moment.VectorialCovarianceValue;
import org.apache.commons.math3.stat.descriptive.moment.VectorialVarianceValue;
import org.apache.commons.math3.stat.descriptive.moment.VectorialSkewnessValue;
import org.apache.commons.math3.stat.descriptive.moment.VectorialKurtosisValue;
import org.apache.commons.math3.stat.descriptive.moment.VectorialCovarianceMatrixValue;
import org.apache.commons.math3.stat.descriptive.moment.VectorialMeanVectorValue;
import org.apache.commons.math3.stat.descriptive.moment.VectorialCentralMomentValue;
import org.apache.commons.math3.stat.descriptive.moment.VectorialFourthMomentValue;
import org.apache.commons.math3.stat.descriptive.moment.VectorialThirdMomentValue;
import org.apache.commons.math3.stat.descriptive.moment.VectorialSecondMomentValue;
import org.apache.commons.math3.stat.descriptive.moment.VectorialProductValue;
import org.apache.commons.math3.stat.descriptive.moment.VectorialMeanValue;
import org.apache.commons.math3.stat.descriptive.moment.VectorialCovarianceValue;
import org.apache.commons.math3.stat.descriptive.moment.VectorialVarianceValue;
import org.apache.commons.math3.stat.descriptive.moment.VectorialSkewnessValue;
import org.apache.commons.math3.stat.descriptive.moment.VectorialKurtosisValue;
import org.apache.commons.math3.stat.descriptive.moment.VectorialCovarianceMatrixValue;
import org.apache.commons.math3.stat.descriptive.moment.VectorialMeanVectorValue;
import org.apache.commons.math3.stat.descriptive.moment.VectorialCentralMomentValue;
import org.apache.commons.math3.stat.descriptive.moment.VectorialFourthMomentValue;
import org.apache.commons.math3.stat.descriptive.moment.VectorialThirdMomentValue;
import org.apache.commons.math3.stat.descriptive.moment.VectorialSecondMomentValue;
import org.apache.commons.math3.stat.descriptive.moment.VectorialProductValue;
import org.apache.commons.math3.stat.descriptive.moment.VectorialMeanValue;
import org.apache.commons.math3.stat.descriptive.moment.VectorialCovarianceValue;
import org.apache.commons.math3.stat.descriptive.moment.VectorialVarianceValue;
import org.apache.commons.math3.stat.descriptive.moment.VectorialSkewnessValue;
import org.apache.commons.math3.stat.descriptive.moment.VectorialKurtosisValue;
import org.apache.commons.math3.stat.descriptive.moment.VectorialCovarianceMatrixValue;
import org.apache.commons.math3.stat.descriptive.moment.VectorialMeanVectorValue;
import org.apache.commons.math3.stat.descriptive.moment.VectorialCentralMomentValue;
import org.apache.commons.math3.stat.descriptive.moment.VectorialFourthMomentValue;
import org.apache.commons.math3.stat.descriptive.moment.VectorialThirdMomentValue;
import org.apache.commons.math3.stat.descriptive.moment.VectorialSecondMomentValue;
import org.apache.commons.math3.stat.descriptive.moment.VectorialProductValue;
import org.apache.commons.math3.stat.descriptive.moment.VectorialMeanValue;
import org.apache.commons.math3.stat.descriptive.moment.VectorialCovarianceValue;
import org.apache.commons.math3.stat.descriptive.moment.VectorialVarianceValue;
import org.apache.commons.math3.stat.descriptive.moment.VectorialSkewnessValue;
import org.apache.commons.math3.stat.descriptive.moment.VectorialKurtosisValue;
import org.apache.commons.math3.stat.descriptive.moment.VectorialCovarianceMatrixValue;
import org.apache.commons.math3.stat.descriptive.moment.VectorialMeanVectorValue;
import org.apache.commons.math3.stat.descriptive.moment.VectorialCentralMomentValue;
import org.apache.commons.math3.stat.descriptive.moment.VectorialFourthMomentValue;
import org.apache.commons.math3.stat.descriptive.moment.VectorialThirdMomentValue;
import org.apache.commons.math3.stat.descriptive.moment.VectorialSecondMomentValue;
import org.apache.commons.math3.stat.descriptive.moment.VectorialProductValue;
import org.apache.commons.math3.stat.descriptive.moment.VectorialMeanValue;
import org.apache.commons.math3.stat.descriptive.moment.VectorialCovarianceValue;
import org.apache.commons.math3.stat.descriptive.moment.VectorialVarianceValue;
import org.apache.commons.math3.stat.descriptive.moment.VectorialSkewnessValue;
import org.apache.commons.math3.stat.descriptive.moment.VectorialKurtosisValue;
import org.apache.commons.math3.stat.descriptive.moment.VectorialCovarianceMatrixValue;
import org.apache.commons.math3.stat.descriptive.moment.VectorialMeanVectorValue;
import org.apache.commons.math3.stat.descriptive.moment.VectorialCentralMomentValue;
import org.apache.commons.math3.stat.descriptive.moment.VectorialFourthMomentValue;
import org.apache.commons.math3.stat.descriptive.moment.VectorialThirdMomentValue;
import org.apache.commons.math3.stat.descriptive.moment.VectorialSecondMomentValue;
import org.apache.commons.math3.stat.descriptive.moment.VectorialProductValue;
import org.apache.commons.math3.stat.descriptive.moment.VectorialMeanValue;
import org.apache.commons.math3.stat.descriptive.moment.VectorialCovarianceValue;
import org.apache.commons.math3.stat.descriptive.moment.VectorialVarianceValue;
import org.apache.commons.math3.stat.descriptive.moment.VectorialSkewnessValue;
import org.apache.commons.math3.stat.descriptive.moment.VectorialKurtosisValue;
import org.apache.commons.math3.stat.descriptive.moment.VectorialCovarianceMatrixValue;
import org.apache.commons.math3.stat.descriptive.moment.VectorialMeanVectorValue;
import org.apache.commons.math3.stat.descriptive.moment.VectorialCentralMomentValue;
import org.apache.commons.math3.stat.descriptive.moment.VectorialFourthMomentValue;
import org.apache.commons.math3.stat.descriptive.moment.VectorialThirdMomentValue;
import org.apache.commons.math3.stat.descriptive.moment.VectorialSecondMomentValue;
import org.apache.commons.math3.stat.descriptive.moment.VectorialProductValue;
import org.apache.commons.math3.stat.descriptive.moment.VectorialMeanValue;
import org.apache.commons.math3.stat.descriptive.moment.VectorialCovarianceValue;
import org.apache.commons.math3.stat.descriptive.moment.VectorialVarianceValue;
import org.apache.commons.math3.stat.descriptive.moment.VectorialSkewnessValue;
import org.apache.commons.math3.stat.descriptive.moment.VectorialKurtosisValue;
import org.apache.commons.math3.stat.descriptive.moment.VectorialCovarianceMatrixValue;
import org.apache.commons.math3.stat.descriptive.moment.VectorialMeanVectorValue;
import org.apache.commons.math3.stat.descriptive.moment.VectorialCentralMomentValue;
import org.apache.commons.math3.stat.descriptive.moment.VectorialFourthMomentValue;
import org.apache.commons.math3.stat.descriptive.moment.VectorialThirdMomentValue;
import org.apache.commons.math3.stat.descriptive.moment.VectorialSecondMomentValue;
import org.apache.commons.math3.stat.descriptive.moment.VectorialProductValue;
import org.apache.commons.math3.stat.descriptive.moment.VectorialMeanValue;
import org.apache.commons.math3.stat.descriptive.moment.VectorialCovarianceValue;
import org.apache.commons.math3.stat.descriptive.moment.VectorialVarianceValue;
import org.apache.commons.math3.stat.descriptive.moment.VectorialSkewnessValue;
import org.apache.commons.math3.stat.descriptive.moment.VectorialKurtosisValue;
import org.apache.commons.math3
阅读全文
相关推荐
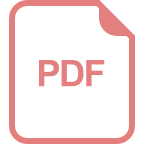
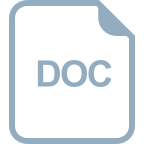
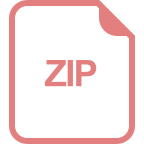
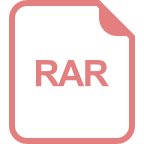
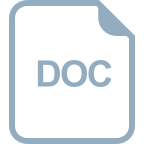
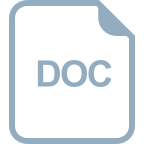
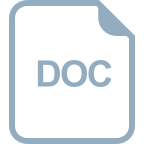

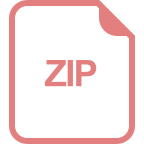
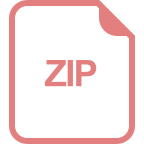
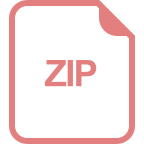
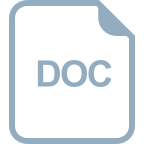
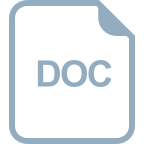


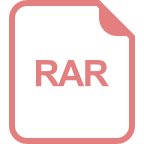
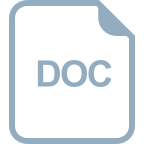