unordered_map的find方法
时间: 2023-12-02 17:43:14 浏览: 44
unordered_map是C++ STL中的一个关联容器,它提供了一种将键映射到值的方法。unordered_map的find方法用于在容器中查找指定键的元素,其函数形式为:unordered_map变量名.find(要查找的键值)。如果找到了指定键的元素,则返回指向该元素的迭代器;否则,返回指向unordered_map尾部的迭代器end()。下面是一个例子:
假设我们有一个unordered_map<int, string> map,其中包含以下键值对:{1: "one", 2: "two", 3: "three"}。我们可以使用find方法查找键为2的元素:
```c++
unordered_map<int, string> map = {{1, "one"}, {2, "two"}, {3, "three"}};
auto it = map.find(2);
if (it != map.end()) {
cout << "Found element with key " << it->first << " and value " << it->second << endl;
} else {
cout << "Element not found" << endl;
}
```
输出结果为:
```
Found element with key 2 and value two
```
相关问题
c unordered_map find
C++中的unordered_map是一种关联容器,它提供了一种键值对的映射关系。unordered_map中的元素是无序的,根据键值进行快速查找。unordered_map的find函数用于在容器中查找指定键的元素。
unordered_map的find函数的使用方式如下:
```cpp
iterator find(const key_type& key);
```
其中,key_type表示键的类型,find函数返回一个迭代器,指向找到的元素,如果未找到,则返回指向unordered_map末尾的迭代器end()。
下面是一个示例代码,演示了如何使用unordered_map的find函数:
```cpp
#include <iostream>
#include <unordered_map>
int main() {
std::unordered_map<int, std::string> myMap = {
{1, "apple"},
{2, "banana"},
{3, "orange"}
};
// 查找键为2的元素
auto it = myMap.find(2);
if (it != myMap.end()) {
std::cout << "Key found! Value: " << it->second << std::endl;
} else {
std::cout << "Key not found!" << std::endl;
}
return 0;
}
```
输出结果为:
```
Key found! Value: banana
```
unordered_map find
unordered_map is a container in C++ that stores elements in an unordered manner based on a hash function. To find an element in an unordered_map, you can use the find() function. Here's an example:
```cpp
#include <iostream>
#include <unordered_map>
int main() {
std::unordered_map<int, std::string> map = {{1, "apple"}, {2, "banana"}, {3, "orange"}};
auto it = map.find(2);
if (it != map.end()) {
std::cout << "Key found! Value is: " << it->second << std::endl;
} else {
std::cout << "Key not found! << std::endl;
}
return 0;
}
```
In this example, we create an unordered_map called `map` with integer keys and string values. We then use the find() function to search for the key 2. If the key is found, it returns an iterator pointing to the key-value pair, and we can access the value using `it->second`. If the key is not found, it returns `map.end()`.
Hope this helps! Let me know if you have any more questions.
相关推荐
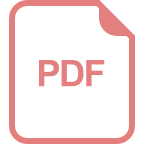
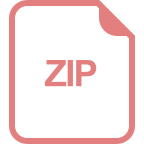












