java使用socket实现tcp协议的excel文件从服务器的上传和下载到本机要有服务器端和上传客户端和下载客户端,并可以把路径a的内容传到路径b把代码写出来并写全
时间: 2024-10-16 20:20:47 浏览: 40
在Java中使用Socket实现TCP协议的Excel文件上传和下载涉及到网络编程的基本操作,包括创建服务器、编写客户端以及处理文件传输。这里我会给出一个简化的示例,但请注意实际项目中需要更完善的错误处理和异常捕获。
首先,我们分别构建服务器端(Server)和两个客户端(UploaderClient 和 DownloaderClient):
**服务器端(Server.java)**
```java
import java.io.*;
import java.net.*;
public class Server {
private ServerSocket serverSocket;
private String serverPath = "D:\\path\\to\\server";
public static void main(String[] args) throws IOException {
new Server().start();
}
public void start() throws IOException {
serverSocket = new ServerSocket(8080); // 设置监听端口
while (true) {
Socket clientSocket = serverSocket.accept(); // 接受连接
new Thread(new FileHandler(clientSocket)).start(); // 开启新线程处理请求
}
}
private class FileHandler implements Runnable {
private Socket socket;
public FileHandler(Socket s) {
this.socket = s;
}
@Override
public void run() {
try {
DataInputStream in = new DataInputStream(socket.getInputStream());
DataOutputStream out = new DataOutputStream(socket.getOutputStream());
String action = in.readUTF(); // 读取命令(upload 或 download)
if ("upload".equals(action)) {
uploadFile(in, out);
} else if ("download".equals(action)) {
downloadFile(out);
} else {
System.out.println("Unknown command");
}
socket.close();
} catch (IOException e) {
e.printStackTrace();
}
}
private void uploadFile(DataInputStream in, DataOutputStream out) {
String srcPath = "/path/to/upload/file.xlsx";
FileInputStream fis = new FileInputStream(serverPath + srcPath);
byte[] buffer = new byte[4096];
int len;
out.writeUTF(srcPath); // 写入目标文件名
while ((len = fis.read(buffer)) > 0) {
out.write(buffer, 0, len);
}
fis.close();
out.flush();
}
private void downloadFile(DataOutputStream out) {
String dstPath = "path/to/download/file.xlsx";
FileOutputStream fos = new FileOutputStream(dstPath);
out.writeUTF(dstPath); // 写入目标文件名
fos.getChannel().transferFrom(
serverSocket.getInputStream(),
0,
Integer.MAX_VALUE); // 从服务器复制数据到本地
fos.close();
}
}
}
```
**上传客户端(UploaderClient.java)**
```java
import java.io.*;
import java.net.*;
public class UploaderClient {
public static void main(String[] args) {
String filePath = "file_to_upload.xlsx";
try (BufferedInputStream bis = new BufferedInputStream(new FileInputStream(filePath))) {
uploadFileToServer(bis, "http://localhost:8080");
} catch (FileNotFoundException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}
}
public static void uploadFileToServer(InputStream inputStream, String url) throws IOException {
URLConnection connection = new URL(url).openConnection();
connection.setDoOutput(true);
OutputStream outputStream = connection.getOutputStream();
DataOutputStream dos = new DataOutputStream(outputStream);
dos.writeUTF("upload"); // 请求上传
dos.writeUTF(filePath); // 写入文件名
byte[] buffer = new byte[1024];
int length;
while ((length = inputStream.read(buffer)) != -1) {
dos.write(buffer, 0, length);
}
dos.flush();
dos.close();
outputStream.close();
}
}
```
**下载客户端(DownloaderClient.java)**
```java
import java.io.*;
import java.net.*;
public class DownloaderClient {
public static void main(String[] args) {
String fileName = "file_to_download.xlsx";
try {
downloadFileFromServer(fileName, "http://localhost:8080");
} catch (IOException e) {
e.printStackTrace();
}
}
public static void downloadFileFromServer(String fileName, String url) throws IOException {
URLConnection connection = new URL(url).openConnection();
InputStream inputStream = connection.getInputStream();
saveToFile(inputStream, fileName);
}
public static void saveToFile(InputStream is, String filename) throws IOException {
FileOutputStream fos = new FileOutputStream(filename);
byte[] buffer = new byte[1024];
int read;
while ((read = is.read(buffer)) != -1) {
fos.write(buffer, 0, read);
}
fos.close();
}
}
```
请注意,上述代码简化了处理过程,实际应用中可能需要添加更多的错误检查、异常处理和数据校验。运行时请确保所有文件路径和URL正确,并按照需求修改`uploadFile`和`downloadFile`方法中的文件路径。
阅读全文
相关推荐
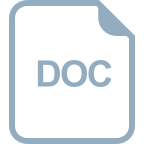
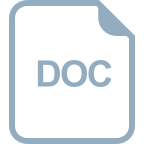
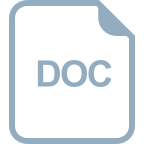
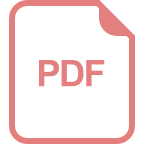
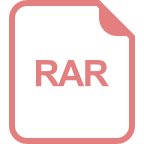
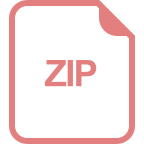
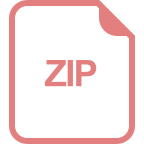
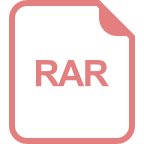
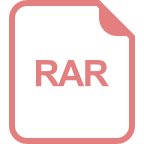
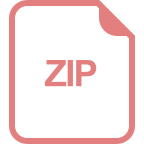
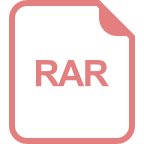
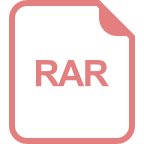
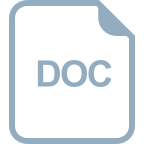
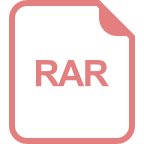
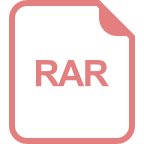
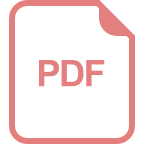
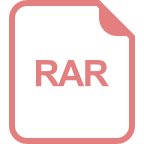
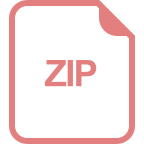