哈希表c语言代码实现
时间: 2024-08-14 17:10:09 浏览: 59
哈希表(也称为散列表)是一种数据结构,通过哈希函数将键映射到数组的特定位置,使得查找、插入和删除操作能够快速完成。下面是一个简单的 C 语言实现哈希表的例子,使用链地址法解决哈希冲突:
```c
#include <stdio.h>
#include <stdlib.h>
#define TABLE_SIZE 10
typedef struct Node {
int key;
int value;
struct Node* next;
} Node;
Node** createHashTable() {
Node** hashTable = (Node**)malloc(sizeof(Node*) * TABLE_SIZE);
for (int i = 0; i < TABLE_SIZE; i++) {
hashTable[i] = NULL;
}
return hashTable;
}
void insert(Node** hashTable, int key, int value) {
int index = hash(key, TABLE_SIZE); // 哈希函数计算索引
Node* newNode = (Node*)malloc(sizeof(Node));
newNode->key = key;
newNode->value = value;
newNode->next = hashTable[index];
hashTable[index] = newNode;
}
int search(Node** hashTable, int key) {
int index = hash(key, TABLE_SIZE);
Node* current = hashTable[index];
while (current != NULL) {
if (current->key == key)
return current->value;
current = current->next;
}
return -1; // 如果未找到,返回 -1 或者抛异常
}
// 简单的哈希函数示例,实际应用中应选择更好的哈希函数
int hash(int key, int size) {
return key % size;
}
int main() {
Node** hashTable = createHashTable();
insert(hashTable, 5, "apple");
insert(hashTable, 10, "banana");
printf("Value for key 10 is: %d\n", search(hashTable, 10)); // 输出 "banana"
// 更多操作...
free(hashTable);
return 0;
}
```
阅读全文
相关推荐












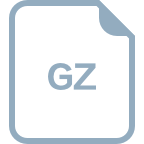





