python读取txt文件事例
时间: 2023-10-31 07:07:37 浏览: 112
这篇文章提供了三种Python读取txt文件的方法,分别是一次性读取、逐行读取和使用fileinput模块。其中一次性读取是最方便的方法,可以将文件中的所有内容一次性读取到一个字符串中。逐行读取方法使用readline()函数,可以一行一行地读取文件内容,虽然比较慢但很省内存。fileinput模块可以同时读取多个文件,并且可以在读取时进行一些处理。具体的代码实现可以参考引用中的三个例子。
相关问题
python读取xml文件代码事例
以下是一个使用Python读取XML文件的示例代码,假设我们有一个名为example.xml的XML文件:
```xml
<?xml version="1.0" encoding="UTF-8"?>
<catalog>
<book id="bk101">
<author>Gambardella, Matthew</author>
<title>XML Developer's Guide</title>
<genre>Computer</genre>
<price>44.95</price>
<publish_date>2000-10-01</publish_date>
<description>An in-depth look at creating applications
with XML.</description>
</book>
<book id="bk102">
<author>Ralls, Kim</author>
<title>Midnight Rain</title>
<genre>Fantasy</genre>
<price>5.95</price>
<publish_date>2000-12-16</publish_date>
<description>A former architect battles corporate zombies,
an evil sorceress, and her own childhood to become queen
of the world.</description>
</book>
</catalog>
```
现在,我们可以使用Python的xml.etree.ElementTree库来读取这个XML文件。以下是一个示例代码:
```python
import xml.etree.ElementTree as ET
# 解析XML文件并获取根元素
tree = ET.parse('example.xml')
root = tree.getroot()
# 遍历XML文件中的元素并访问它们的属性和文本
for child in root:
print('Book ID:', child.attrib['id'])
print('Author:', child.find('author').text)
print('Title:', child.find('title').text)
print('Genre:', child.find('genre').text)
print('Price:', child.find('price').text)
print('Publish Date:', child.find('publish_date').text)
print('Description:', child.find('description').text)
print()
# 使用XPath表达式来选择XML文档中的元素
for elem in root.findall('.//book[genre="Fantasy"]'):
print('Fantasy Book ID:', elem.attrib['id'])
print('Author:', elem.find('author').text)
print('Title:', elem.find('title').text)
print()
```
在上面的示例代码中,我们首先使用ET.parse()函数解析XML文件,并使用getroot()方法获取根元素。然后,我们使用for循环遍历XML文件中的每个book元素,并使用find()方法访问其子元素的文本内容。最后,我们使用XPath表达式".//book[genre="Fantasy"]"来选择所有genre为"Fantasy"的book元素,并输出它们的属性和文本内容。
请举例python迭代器读取文件的事例
下面是一个 Python 迭代器读取文件的例子:
```python
class FileReader:
def __init__(self, filename):
self.filename = filename
def __iter__(self):
with open(self.filename) as f:
for line in f:
yield line.strip()
# 使用迭代器读取文件
for line in FileReader('example.txt'):
print(line)
```
在上面的代码中,我们创建了一个名为 `FileReader` 的类,该类接受一个文件名作为参数并返回一个迭代器。在 `__iter__` 方法中,我们打开文件并使用 `yield` 关键字返回每一行的内容。然后,我们可以使用 `for` 循环来遍历迭代器并逐行打印文件内容。
阅读全文
相关推荐






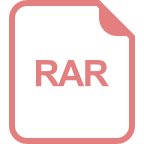









