生成代码 检测一个变量是否发生变化
时间: 2024-10-27 08:13:55 浏览: 41
在编程中,检测一个变量是否发生变化通常涉及到比较变量在不同时间点的值。如果你需要实时监控一个变量的变化,可以采用以下几种方式:
1. **使用观察者模式**:如果你正在使用支持事件驱动的语言(如JavaScript的`Object.observe`、Python的`watchdog`库),可以设置一个观察者监听该变量的更改。当变量值改变时,会触发相应的回调函数。
```python
import watchdog.observers
import watchdog.events
class ValueObserver(watchdog.events.FileSystemEventHandler):
def on_modified(self, event):
if event.src_path == 'my_variable':
print('Variable has changed')
observer = watchdog.observers.Observer()
observer.schedule(ValueObserver(), path='.')
observer.start()
# ... later, modify my_variable
```
2. **使用属性监听工具**:某些编程环境提供特定的API或库,比如Kotlin的`PropertyChangeSupport`,用于检测属性变化。
```kotlin
class MyClass {
private var value: String by PropertyChangeSupport()
// ... set and get value methods
fun onChanged() {
println("Value has changed to $value")
}
fun setValue(newValue: String) {
val oldValue = this.value
this.value = newValue
notifyListeners(ValueChangeEvent(this, oldValue, newValue))
}
}
```
3. **手动比较**:在一些不支持直接监听的环境中,你可以选择定期检查变量,例如每隔一段时间做一次对比。
```java
public class MyVariableMonitor {
private String currentValue;
public void checkForChanges() {
if (!currentValue.equals(lastCheckedValue)) {
System.out.println("Variable has changed from " + lastCheckedValue + " to " + currentValue);
}
lastCheckedValue = currentValue;
// schedule the next check after a delay
}
// ... update currentValue as needed
}
```
阅读全文
相关推荐





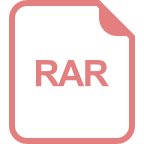






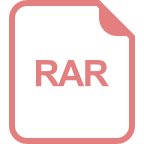





