tensorflow图像去雾算法代码
时间: 2024-01-23 18:00:45 浏览: 110
TensorFlow中常用的图像去雾算法包括Retinex去雾算法和DeepDehazing深度去雾算法。以下是一个简单的TensorFlow图像去雾算法代码示例:
```python
import tensorflow as tf
from tensorflow.keras import layers
from tensorflow.keras import Model
# 定义Retinex去雾模型
class RetinexDehazing(Model):
def __init__(self):
super(RetinexDehazing, self).__init__()
# 定义卷积层
self.conv1 = layers.Conv2D(32, (3, 3), activation='relu', padding='same')
self.conv2 = layers.Conv2D(64, (3, 3), activation='relu', padding='same')
self.conv3 = layers.Conv2D(3, (3, 3), activation='linear', padding='same')
def call(self, inputs):
# 进行图像去雾处理
x = self.conv1(inputs)
x = self.conv2(x)
x = self.conv3(x)
return x
# 定义深度去雾模型
class DeepDehazing(Model):
def __init__(self):
super(DeepDehazing, self).__init__()
# 定义卷积层和残差连接
self.conv1 = layers.Conv2D(32, (3, 3), activation='relu', padding='same')
self.conv2 = layers.Conv2D(64, (3, 3), activation='relu', padding='same')
self.conv3 = layers.Conv2D(3, (3, 3), activation='linear', padding='same')
def call(self, inputs):
# 进行图像去雾处理
x = self.conv1(inputs)
x = self.conv2(x)
x = tf.add(x, inputs) # 残差连接
x = self.conv3(x)
return x
# 读取输入图像
input_image = tf.io.read_file('input.jpg')
input_image = tf.image.decode_jpeg(input_image, channels=3)
input_image = tf.image.convert_image_dtype(input_image, tf.float32)
input_image = tf.expand_dims(input_image, 0)
# 使用Retinex去雾模型
retinex_model = RetinexDehazing()
output_image_retinex = retinex_model(input_image)
# 使用DeepDehazing去雾模型
deep_model = DeepDehazing()
output_image_deep = deep_model(input_image)
# 显示处理后的图像
import matplotlib.pyplot as plt
plt.figure(figsize=(8, 8))
plt.subplot(1, 2, 1)
plt.title('Retinex Dehazing')
plt.imshow(output_image_retinex[0].numpy())
plt.axis('off')
plt.subplot(1, 2, 2)
plt.title('Deep Dehazing')
plt.imshow(output_image_deep[0].numpy())
plt.axis('off')
plt.show()
```
阅读全文
相关推荐
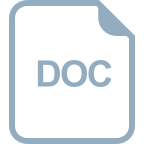
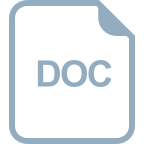
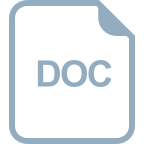
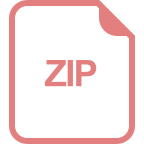


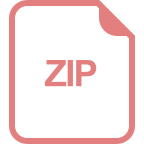
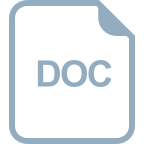



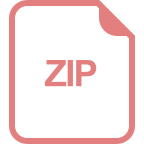
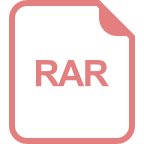
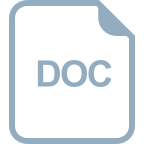

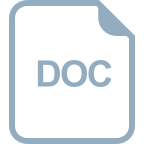
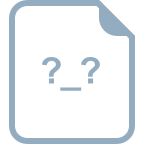
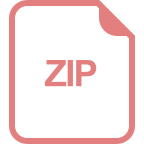