ios firebase消息推送
时间: 2025-01-02 10:38:20 浏览: 8
### iOS Firebase 消息推送实现教程
#### 创建 Firebase 项目并配置
为了在 iOS 平台上使用 Firebase 进行消息推送,需先创建一个 Firebase 项目并将此项目与 iOS 应用关联[^1]。
#### 下载 `GoogleService-Info.plist` 文件
完成 Firebase 项目的创建之后,在 Firebase 控制台中下载 `GoogleService-Info.plist` 文件,并将其添加至 Xcode 工程内。该文件包含了连接应用到 Firebase 所必需的信息[^2]。
#### 配置 Podfile 和安装依赖项
确保 CocoaPods 安装完毕后,在项目的根目录下找到或创建名为 `Podfile` 的文件,向其中加入如下内容:
```ruby
target 'YourTargetName' do
pod 'Firebase/Messaging'
end
```
执行命令来更新 Pods:
```bash
pod install
```
#### 初始化 Firebase SDK
打开 `.xcworkspace` 文件启动项目,在应用程序入口处导入 Firebase 并初始化 SDK:
```swift
import UIKit
import Firebase
@main
class AppDelegate: UIResponder, UIApplicationDelegate {
func application(_ application: UIApplication,
didFinishLaunchingWithOptions launchOptions: [UIApplication.LaunchOptionsKey: Any]?) -> Bool {
FirebaseApp.configure()
// 注册远程通知支持
if #available(iOS 10.0, *) {
let authOptions: UNAuthorizationOptions = [.alert, .badge, .sound]
UNUserNotificationCenter.current().requestAuthorization(
options: authOptions,
completionHandler: {_, _ in })
Messaging.messaging().delegate = self as? MessagingDelegate
} else {
let settings: UIUserNotificationSettings =
UIUserNotificationSettings(types: [.alert, .badge, .sound], categories: nil)
application.registerUserNotificationSettings(settings)
}
application.registerForRemoteNotifications()
return true
}
}
```
#### 处理接收的消息
当设备接收到一条推送通知时,可以通过实现代理方法处理这些事件。对于前台运行的应用程序来说尤为重要,因为默认情况下只有当应用处于后台时才会显示通知。
```swift
extension AppDelegate : MessagingDelegate {
/// 收到来自 FCM 的新令牌 (用于发送目标化信息)
func messaging(_ messaging: Messaging, didReceiveRegistrationToken fcmToken: String?) {
print("Firebase registration token: \(String(describing: fcmToken))")
}
/// 当应用正在活跃状态时收取消息
func userNotificationCenter(_ center: UNUserNotificationCenter,
willPresent notification: UNNotification,
withCompletionHandler completionHandler: @escaping (UNNotificationPresentationOptions) -> Void) {
completionHandler([.alert, .badge, .sound])
}
}
// MARK: - Remote Notification Handling Methods
extension AppDelegate {
func application(_ application: UIApplication,
didRegisterForRemoteNotificationsWithDeviceToken deviceToken: Data) {
Messaging.messaging().apnsToken = deviceToken
}
}
```
通过上述步骤可以成功地将 Firebase Cloud Messaging 整合进 iOS 应用当中,从而允许开发者利用 Firebase 提供的服务来进行高效可靠的通知传递服务。
阅读全文
相关推荐











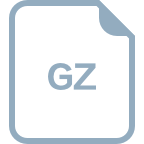






