C语言在键盘中输入两个字符串,“happy”,“everyday”,求每个字符串的长度、把长的字符串联结到短的之后、把短的字符串变味的大写。
时间: 2024-12-22 15:21:30 浏览: 5
在C语言中,你可以通过以下几个步骤来实现这个功能:
1. **获取字符串长度**:可以使用`strlen()`函数分别计算两个字符串"happy"和"everyday"的长度。
```c
#include <stdio.h>
#include <string.h>
int main() {
char str1[] = "happy";
char str2[] = "everyday";
int len1 = strlen(str1);
int len2 = strlen(str2);
printf("Length of 'happy': %d\n", len1);
printf("Length of 'everyday': %d\n", len2);
}
```
2. **连接两个字符串**:如果`len1 > len2`,则将`str2`追加到`str1`的后面;反之,则将`str1`追加到`str2`后面。这里需要额外的空间存储结果,你可以先复制较短的那个字符串到新的数组里,然后再追加另一个。
```c
char combined[50]; // 假设最大长度为48 (50 - 2 for null termination)
if(len1 > len2) {
strcpy(combined, str1);
strcat(combined, str2);
} else {
strcpy(combined, str2);
strcat(combined, str1);
}
printf("Combined string: %s\n", combined);
```
3. **转换小写的字符串**:最后,将短的字符串转为大写。注意,在C标准库中,直接修改字符串可能会导致问题,因为字符串实际上是字符数组,不是真正的可变对象。所以通常会先创建一个新的临时字符串。这里是例子,假设`shortStr`是指向`str2`的指针:
```c
char shortUpperCase[50];
strcpy(shortUpperCase, str2); // Copy the short string
for(int i = 0; i < len2; i++) {
shortUpperCase[i] = toupper(shortUpperCase[i]);
}
printf("Short string in uppercase: %s\n", shortUpperCase);
```
阅读全文
相关推荐
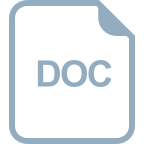
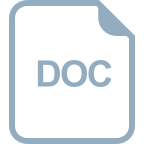
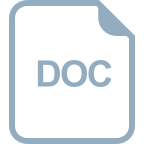
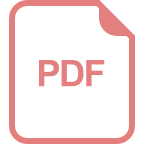
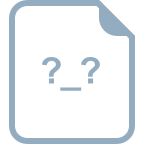
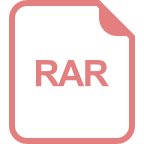
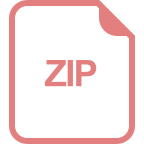
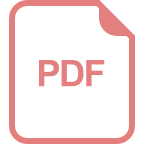
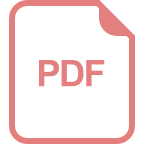
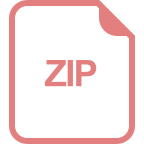
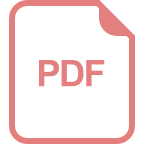
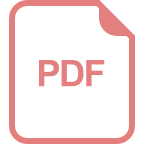