eclipse,spring boot框架下,使用DataVec 读取CSV数据具体代码
时间: 2024-12-02 13:28:24 浏览: 12
Apache DataVec 是一个强大的大数据处理库,它包含了一系列用于转换、清洗和分析数据的工具。在 Spring Boot 框架下使用 DataVec 读取 CSV 数据,你需要先确保已经添加了相关的依赖。以下是一段简单的示例代码,展示了如何在 Eclipse 中进行操作:
1. 添加 Maven 配置(如果你还没有在 `pom.xml` 文件中添加 DataVec 和 Spring Boot 相关依赖):
```xml
<dependencies>
<!-- Other Spring Boot dependencies -->
<dependency>
<groupId>org.apache.datavec</groupId>
<artifactId>datavec-api-spark_2.11</artifactId>
<version>0.13.0</version> <!-- Update to the latest version if needed -->
</dependency>
<dependency>
<groupId>org.apache.spark</groupId>
<artifactId>spark-core_2.11</artifactId>
<version>2.4.5</version> <!-- Update to the Spark version that matches your DataVec dependency -->
</dependency>
<!-- If you use Spring Data Commons for file handling -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-commons</artifactId>
</dependency>
</dependencies>
```
2. 创建一个 Spring Boot 类并引入相关配置:
```java
import org.apache.spark.sql.Dataset;
import org.apache.spark.sql.Row;
import org.apache.spark.sql.SparkSession;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Component;
@Component
public class CSVReader {
@Autowired
private DataVecSparkSession dataVecSparkSession; // Assuming you have an autowired DataVecSparkSession
public Dataset<Row> readCSV(String filePath) {
return dataVecSparkSession.read()
.csv(filePath)
.asType(Double.class); // Assuming you want to load as double values, change accordingly
}
}
```
在这个例子中,我们假设你已经有了一个 `DataVecSparkSession` 的实例(可以通过Spring Boot的自动注入或自定义创建)。`dataVecSparkSession.read().csv(filePath)` 会读取指定路径下的 CSV 文件,`.asType(Double.class)` 表示每一列的数据类型为 `Double`。
3. 在 Spring Boot 主应用中启动 Spark 和 DataVec:
```java
@SpringBootApplication
public class Application {
public static void main(String[] args) {
SpringApplication.run(Application.class, args);
// Start the Spark and DataVec contexts (assuming a method like this exists in your application)
startSparkAndDataVec();
}
// Method to start Spark and DataVec contexts
private static void startSparkAndDataVec() {
// Configure and start Spark session here
SparkConf sparkConf = new SparkConf().setAppName("CSVReaderApp");
SparkSession spark = SparkSession.builder().config(sparkConf).getOrCreate();
// Initialize DataVecSparkSession using Spark session
DataVecSparkSession.init(spark);
}
}
```
记得根据实际项目需求调整文件路径、数据类型和 Spark 配置。
阅读全文
相关推荐
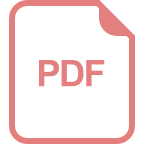
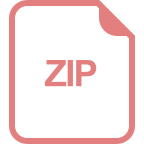
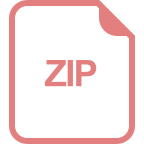
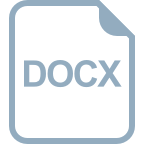
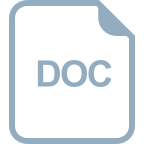