基于LinkedHashMap实现LRU算法
时间: 2023-12-25 15:25:12 浏览: 102
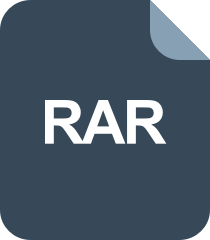
一个LRU算法的实现
基于LinkedHashMap实现LRU算法的核心是继承LinkedHashMap类,并设置accessOrder参数为true,这样可以按照访问顺序对元素进行排序。同时,需要重写removeEldestEntry()方法来控制缓存的大小,当缓存满时删除最老的元素。
以下是基于LinkedHashMap实现LRU算法的代码示例:
```java
import java.util.LinkedHashMap;
import java.util.Map;
public class LRUCache<K, V> extends LinkedHashMap<K, V> {
private int capacity;
public LRUCache(int capacity) {
super(16, 0.75f, true);
this.capacity = capacity;
}
@Override
protected boolean removeEldestEntry(Map.Entry<K, V> eldest) {
return size() > capacity;
}
}
// 使用示例
public static void main(String[] args) {
LRUCache<String, Integer> cache = new LRUCache<>(3);
cache.put("1", 1);
cache.put("2", 2);
cache.put("3", 3);
System.out.println(cache.get("1")); // 输出1
cache.put("4", 4);
System.out.println(cache.get("2")); // 输出null
cache.put("5", 5);
System.out.println(cache.containsKey("3")); // 输出false
cache.put("6", 6);
// 输出缓存中的键值对
cache.forEach((k, v) -> System.out.println(k + ":" + v));
// 输出6:6 4:4 5:5
System.out.println(cache.size()); // 输出3
}
```
阅读全文
相关推荐
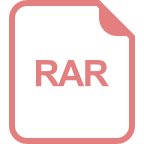

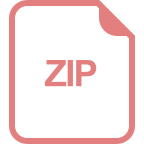
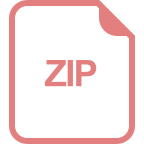
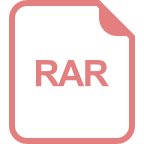
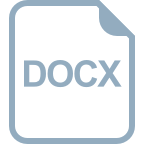
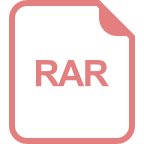
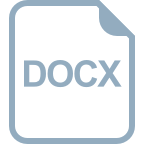
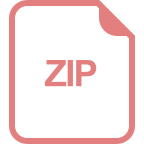
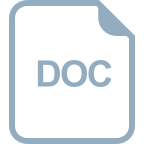
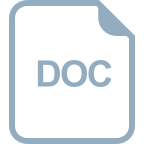
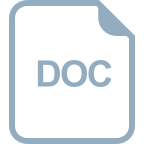
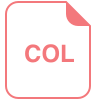



