python绘制五星红旗
时间: 2024-09-10 18:00:47 浏览: 21
在Python中,你可以使用`matplotlib`这个库来绘制五星红旗。`matplotlib`是一个用于创建静态、动画和交互式可视化的库。五星红旗的绘制涉及到红色背景上绘制一个大的五角星和四个小的五角星。小五角星需要按照特定的角度和位置排列,以便它们看起来像是围绕着大五角星。
以下是一个简化的代码示例,描述了如何使用Python的`matplotlib`库绘制五星红旗:
```python
import matplotlib.pyplot as plt
import numpy as np
def draw_star(center, radius, color, ax):
"""绘制五角星的函数"""
x = center[0]
y = center[1]
# 生成五角星五个顶点的坐标
pts = []
for i in range(5):
x1 = x + radius * np.cos((18 + i * 72) / 180 * np.pi)
y1 = y + radius * np.sin((18 + i * 72) / 180 * np.pi)
pts.append((x1, y1))
x2 = x + radius * np.cos((54 + i * 72) / 180 * np.pi) * np.cos(18 / 180 * np.pi)
y2 = y + radius * np.sin((54 + i * 72) / 180 * np.pi) * np.cos(18 / 180 * np.pi)
pts.append((x2, y2))
# 连接顶点绘制五角星
star = plt.Polygon(pts, color=color)
ax.add_patch(star)
# 创建绘图的画布
fig, ax = plt.subplots(figsize=(10, 7))
ax.set_xlim(0, 30)
ax.set_ylim(0, 20)
# 绘制红色背景
ax.add_patch(plt.Rectangle((0, 0), 30, 20, color='red'))
# 绘制大星星
draw_star(center=(5, 15), radius=3, color='yellow', ax=ax)
# 绘制四个小星星
draw_star(center=(10, 18), radius=1, color='yellow', ax=ax)
draw_star(center=(12, 16), radius=1, color='yellow', ax=ax)
draw_star(center=(12, 13), radius=1, color='yellow', ax=ax)
draw_star(center=(10, 11), radius=1, color='yellow', ax=ax)
# 隐藏坐标轴
ax.axis('off')
# 显示图像
plt.show()
```
这段代码定义了一个绘制五角星的函数`draw_star`,它接受中心点坐标、半径、颜色和轴对象作为参数。然后,代码设置了画布、绘制红色背景,并使用`draw_star`函数绘制了一个大星星和四个小星星。
相关推荐
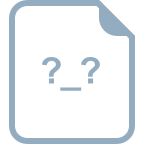
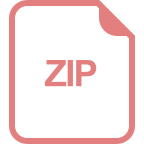
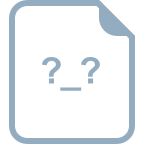












