从京东爬取商品的评论,进行情感类别标注,并抽取情感词汇 python代码
时间: 2024-02-11 21:25:22 浏览: 81
要从京东爬取商品评论并进行情感类别标注以及抽取情感词汇,你可以使用Python中的一些爬虫和自然语言处理工具。下面是一个示例代码,使用requests库进行网页请求,使用BeautifulSoup库进行网页解析,然后使用NLTK库进行情感类别标注和情感词汇抽取:
```python
import requests
from bs4 import BeautifulSoup
import nltk
from nltk.corpus import stopwords
from nltk.tokenize import word_tokenize
from nltk.sentiment import SentimentIntensityAnalyzer
# 下载必要的NLTK数据
nltk.download('vader_lexicon')
nltk.download('stopwords')
nltk.download('punkt')
def extract_sentiment_words(text):
# 初始化情感强度分析器
sid = SentimentIntensityAnalyzer()
# 分词并移除停用词
stop_words = set(stopwords.words('chinese'))
word_tokens = word_tokenize(text)
filtered_tokens = [w for w in word_tokens if not w in stop_words]
# 对每个单词进行情感分析,并抽取情感词汇
sentiment_words = []
for word in filtered_tokens:
sentiment_score = sid.polarity_scores(word)
if sentiment_score['compound'] != 0.0: # 根据情感强度判断是否为情感词汇
sentiment_words.append(word)
return sentiment_words
# 爬取京东商品评论页面
url = 'https://item.jd.com/100008348542.html#comment'
response = requests.get(url)
html = response.text
# 解析评论内容
soup = BeautifulSoup(html, 'html.parser')
comments = soup.find_all('div', class_='comment-item')
# 对每条评论进行情感类别标注和情感词汇抽取
for comment in comments:
comment_text = comment.find('div', class_='comment-con').text.strip()
sentiment_words = extract_sentiment_words(comment_text)
sentiment = 'positive' if len(sentiment_words) > 0 else 'negative'
print(comment_text)
print('Sentiment: ', sentiment)
print('Sentiment words: ', sentiment_words)
print('-----------------------')
```
请注意,这段代码假设你已经通过requests库爬取了京东商品的评论页面,并且评论内容位于`<div class="comment-con">`标签中。代码将使用BeautifulSoup库解析HTML,并使用NLTK库中的`SentimentIntensityAnalyzer`进行情感分析,使用`stopwords`库移除停用词,使用`word_tokenize`函数进行分词。最后,代码将进行情感类别标注和情感词汇抽取,并打印结果。
你可以根据需要修改代码以适应你的具体任务和数据。另外,请确保你在进行网络爬取时遵守相关网站的使用条款和政策。
阅读全文
相关推荐
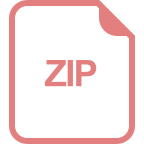
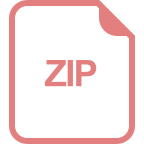
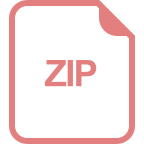
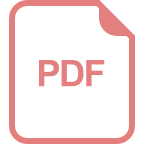
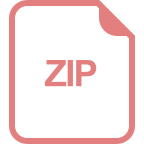
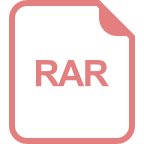
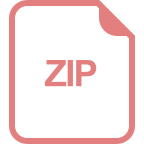
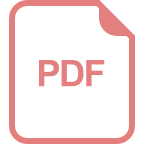
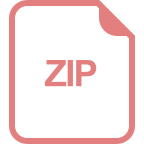
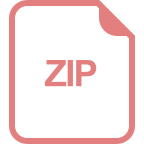
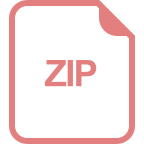
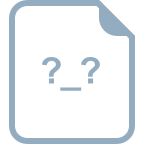
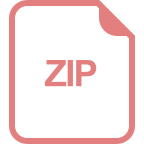
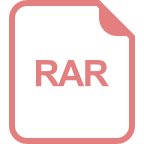
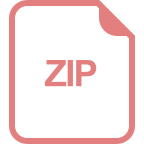
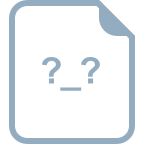
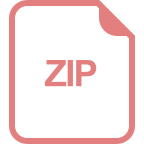