编写一个使用继承语法的案例 1)定义一个父类(水果) 2)定义两个子类(颜色,果型),两个子类必须要继承父类 3)父类里面至少要有三个属性(颜色,果型,味道) 4)父类里面至少要有四种不同类型的方法
时间: 2024-01-31 07:02:49 浏览: 21
以下是一个使用继承语法的案例,父类是"水果",子类是"颜色"和"果型":
```python
class Fruit:
def __init__(self, color, shape, taste):
self.color = color
self.shape = shape
self.taste = taste
def eat(self):
print("吃水果")
def peel(self):
print("剥水果皮")
def describe(self):
print(f"这个水果是{self.color}色的,{self.shape}状的,味道{self.taste}")
class Color(Fruit):
def __init__(self, color, shape, taste):
super().__init__(color, shape, taste)
def change_color(self, new_color):
self.color = new_color
print(f"水果颜色变成了{self.color}")
class Shape(Fruit):
def __init__(self, color, shape, taste):
super().__init__(color, shape, taste)
def change_shape(self, new_shape):
self.shape = new_shape
print(f"水果形状变成了{self.shape}")
# 测试代码
apple = Color("红色", "圆形", "甜")
apple.describe() # 输出:这个水果是红色的,圆形状的,味道甜
apple.eat() # 输出:吃水果
apple.change_color("绿色") # 输出:水果颜色变成了绿色
apple.describe() # 输出:这个水果是绿色的,圆形状的,味道甜
banana = Shape("黄色", "弯曲", "香甜")
banana.describe() # 输出:这个水果是黄色的,弯曲状的,味道香甜
banana.peel() # 输出:剥水果皮
banana.change_shape("直的") # 输出:水果形状变成了直的
banana.describe() # 输出:这个水果是黄色的,直的状的,味道香甜
```
在这个案例中,父类"水果"定义了三个属性(颜色、果型和味道)以及四个方法(吃水果、剥水果皮、描述、具体子类可以添加自己的方法)。子类"颜色"和"果型"分别继承了父类"水果",并添加了自己特有的方法(改变颜色和改变形状)。通过创建实例并调用方法,可以测试父类和子类的功能。
相关推荐
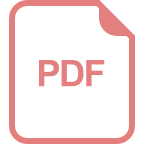

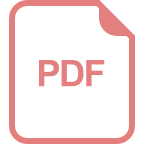
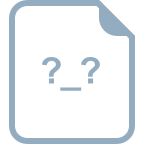
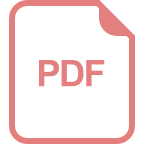
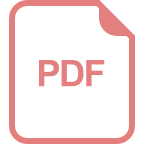
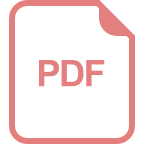
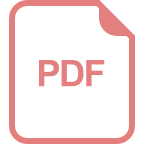
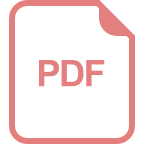
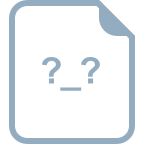
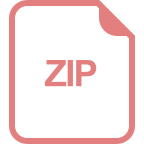
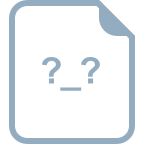
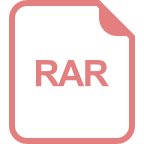