使用【python】写【基于人工智能的自然语言处理系统分析】的代码
时间: 2024-09-10 17:13:49 浏览: 87
编写一个基于人工智能的自然语言处理系统分析的Python代码通常涉及到以下步骤:
1. 数据预处理:包括文本清洗、分词、去除停用词等。
2. 特征提取:将文本数据转换为机器学习模型可以处理的格式,如词袋模型、TF-IDF、Word2Vec等。
3. 模型选择:选择合适的机器学习或深度学习模型进行训练,如朴素贝叶斯、支持向量机、循环神经网络(RNN)、长短时记忆网络(LSTM)或Transformer模型等。
4. 训练模型:使用训练数据对模型进行训练。
5. 模型评估:使用验证集或测试集评估模型的性能。
6. 应用模型:将训练好的模型用于新的数据集进行分析。
下面是一个简单的例子,使用Python中的`nltk`库进行文本预处理,使用`sklearn`库构建TF-IDF特征并训练一个朴素贝叶斯模型进行情感分析:
```python
import nltk
from sklearn.feature_extraction.text import TfidfVectorizer
from sklearn.naive_bayes import MultinomialNB
from sklearn.pipeline import make_pipeline
from sklearn.model_selection import train_test_split
from sklearn.metrics import accuracy_score
# 示例数据集
data = [
"I love this phone",
"This is a bad phone",
"I hate this product",
"It is an amazing product",
"I feel happy with this phone",
"This is a horrible device"
]
labels = [1, 0, 0, 1, 1, 0] # 1代表正面情感,0代表负面情感
# 文本预处理(分词)
nltk.download('punkt')
tokenized_data = [nltk.word_tokenize(text) for text in data]
# 特征提取
tfidf_vectorizer = TfidfVectorizer(stop_words='english')
# 划分训练集和测试集
X_train, X_test, y_train, y_test = train_test_split(tokenized_data, labels, test_size=0.33, random_state=42)
# 创建管道,集成TF-IDF向量化和朴素贝叶斯分类器
model = make_pipeline(TfidfVectorizer(), MultinomialNB())
# 训练模型
model.fit(X_train, y_train)
# 进行预测
predictions = model.predict(X_test)
# 评估模型
print("Accuracy:", accuracy_score(y_test, predictions))
# 使用模型进行预测
new_texts = ["I am satisfied with this service", "This is a terrible experience"]
predicted_labels = model.predict(new_texts)
print(predicted_labels)
```
请注意,这只是一个非常简单的示例,实际应用中可能需要更复杂的预处理步骤,更高级的模型以及更细致的参数调整来达到更好的效果。
阅读全文
相关推荐
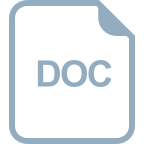
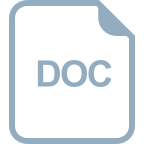
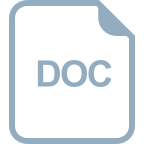
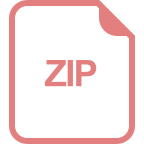
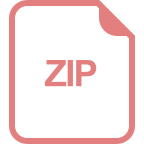
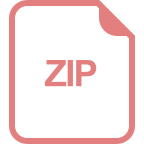
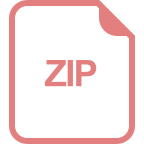
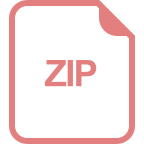
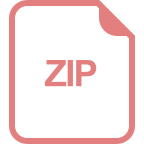
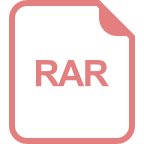
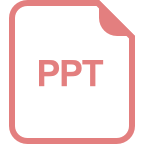
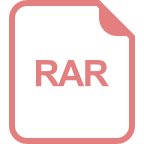
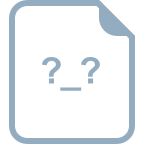
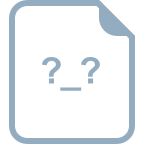
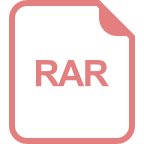
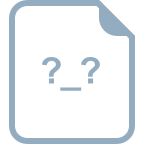
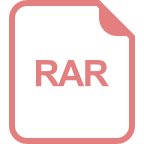
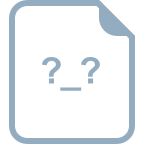
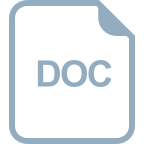