还是刚刚那个问题,要求给出c语言代码
时间: 2024-10-29 07:19:47 浏览: 4
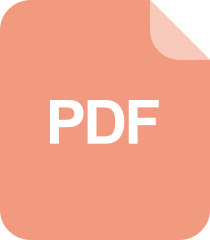
如何写出优美的C语言代码
在C语言中处理这个问题比较复杂,因为PIL这样的图像处理库并不直接适用于C。不过,你可以使用标准库如`uchar`、`gray_scale.c`(用于灰度处理)以及基本的位操作来实现类似的功能。这里提供一个简化版的C代码示例,但请注意,它可能不如Python那样直观易懂,也未包含完整的错误检查:
```c
#include <stdio.h>
#include <stdlib.h>
// 从灰度图片读取并处理红色部分
void process_image(const char* input_path, char* output_path) {
FILE* input_file = fopen(input_path, "rb");
if (!input_file) {
printf("Error opening image file.\n");
return;
}
// 假设输入是单通道的BMP格式,每行像素以字节流的形式存储
int width, height;
fseek(input_file, 14, SEEK_SET); // 跳过前14字节的头信息
fread(&width, sizeof(int), 1, input_file);
fread(&height, sizeof(int), 1, input_file);
// 简化的灰度处理
unsigned char* gray_data = (unsigned char*)malloc(width * height);
fseek(input_file, 18, SEEK_SET); // 跳过位深信息
for (int y = 0; y < height; y++) {
fseek(input_file, y * width, SEEK_CUR);
fread(gray_data + y * width, width, 1, input_file);
}
fclose(input_file);
// 对红通道进行二值化
for (int i = 0; i < width * height; i++) {
gray_data[i] = (gray_data[i] > 0) ? 255 : 0; // 将大于0的灰度值设为白色,小于等于0的设为黑色
}
// 写入新的黑白图像文件
FILE* output_file = fopen(output_path, "wb");
fwrite(gray_data, width * height, 1, output_file);
free(gray_data);
fclose(output_file);
}
int main() {
const char* input_path = "input.bmp";
char* output_path = "output.bmp"; // 输出黑白图片路径
process_image(input_path, output_path);
return 0;
}
```
这只是一个简化的示例,实际使用时可能需要更复杂的图像结构解析和内存管理。记住,C语言对图像处理的支持有限,如果性能要求高或功能复杂,建议使用专门的图像处理库,比如OpenCV或FreeImage等。
阅读全文
相关推荐
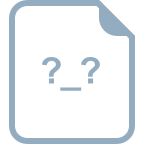
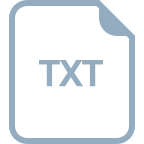