python如何批量替换成一个字符
时间: 2024-09-22 15:02:58 浏览: 34
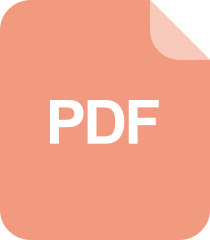
python批量替换文件名中的共同字符实例
在Python中,你可以使用内置的`str.replace()`函数或列表推导式来进行批量替换操作。这里有两个简单的方法:
1. **使用replace()函数**:
```python
text = "Hello, World!"
new_text = text.replace("World", "Python") # 将所有"World"替换成"Python"
print(new_text)
```
2. **使用列表推导式**(如果需要替换的是多个字符或复杂的模式):
```python
import re # 引入正则表达式库
def batch_replace(text, old_chars, new_char):
return ''.join([new_char if char in old_chars else char for char in text])
original = "Hello, World!"
old_chars = ["o", "W"]
new_char = "*"
result = batch_replace(original, old_chars, new_char)
print(result)
```
在这两个例子中,`replace()`会直接替换字符串中所有的匹配项,而列表推导式则可以处理更复杂的查找替换条件。
阅读全文
相关推荐
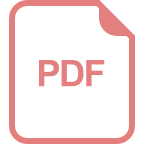
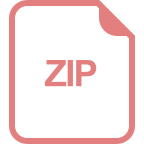
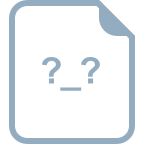
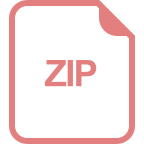
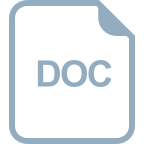









