语义分割和目标检测的区别
时间: 2024-06-13 11:05:47 浏览: 24
语义分割和目标检测是计算机视觉领域中常用的图像分析任务,它们在目标识别和场景理解方面发挥着重要作用。它们的区别如下:
1. 目标检测主要关注物体定位和分类,可以处理多个物体实例;而语义分割注重像素级别的物体分割和类别标记,无法区分不同实例。
2. 目标检测需要在图像中定位物体的位置,并对其进行分类,通常使用边界框来表示物体的位置和大小;而语义分割则需要对图像中的每个像素进行分类,将每个像素标记为属于哪个类别。
3. 目标检测可以处理遮挡、旋转、缩放等情况,而语义分割则无法区分不同实例,对于遮挡、重叠的物体会出现误判。
4. 目标检测可以输出物体的位置和大小信息,适用于需要知道物体具体位置的应用场景;而语义分割则更适用于需要对图像进行像素级别的分析和理解的场景。
下面是一个目标检测和语义分割的示例代码:
```python
# 目标检测示例代码
import cv2
# 加载模型和标签
net = cv2.dnn.readNetFromDarknet('yolov3.cfg', 'yolov3.weights')
classes = []
with open('coco.names', 'r') as f:
classes = [line.strip() for line in f.readlines()]
# 加载图像
img = cv2.imread('dog.jpg')
# 对图像进行目标检测
blob = cv2.dnn.blobFromImage(img, 1/255.0, (416, 416), swapRB=True, crop=False)
net.setInput(blob)
layer_names = net.getLayerNames()
output_layers = [layer_names[i[0]-1] for i in net.getUnconnectedOutLayers()]
outs = net.forward(output_layers)
# 处理检测结果
class_ids = []
confidences = []
boxes = []
for out in outs:
for detection in out:
scores = detection[5:]
class_id = np.argmax(scores)
confidence = scores[class_id]
if confidence > 0.5:
center_x = int(detection[0] * img.shape[1])
center_y = int(detection[1] * img.shape[0])
w = int(detection[2] * img.shape[1])
h = int(detection[3] * img.shape[0])
x = int(center_x - w/2)
y = int(center_y - h/2)
class_ids.append(class_id)
confidences.append(float(confidence))
boxes.append([x, y, w, h])
# 绘制检测结果
indexes = cv2.dnn.NMSBoxes(boxes, confidences, 0.5, 0.4)
font = cv2.FONT_HERSHEY_PLAIN
colors = np.random.uniform(0, 255, size=(len(classes), 3))
for i in range(len(boxes)):
if i in indexes:
x, y, w, h = boxes[i]
label = str(classes[class_ids[i]])
color = colors[class_ids[i]]
cv2.rectangle(img, (x, y), (x+w, y+h), color, 2)
cv2.putText(img, label, (x, y-5), font, 1, color, 2)
# 显示结果
cv2.imshow('Image', img)
cv2.waitKey(0)
cv2.destroyAllWindows()
# 语义分割示例代码
import cv2
# 加载模型和标签
net = cv2.dnn.readNetFromTorch('deeplabv3_1.t7')
classes = []
with open('labels.txt', 'r') as f:
classes = [line.strip() for line in f.readlines()]
# 加载图像
img = cv2.imread('dog.jpg')
# 对图像进行语义分割
blob = cv2.dnn.blobFromImage(img, 1/255.0, (513, 513), swapRB=True, crop=False)
net.setInput(blob)
out = net.forward()
# 处理分割结果
out = out[0, :, :, :]
out = cv2.resize(out, (img.shape[1], img.shape[0]))
out = np.argmax(out, axis=2)
# 绘制分割结果
mask = np.zeros((img.shape[0], img.shape[1], 3), dtype=np.uint8)
for i in range(len(classes)):
mask[out == i] = np.array([0, 0, 255]) # 将每个类别的像素标记为红色
result = cv2.addWeighted(img, 0.5, mask, 0.5, 0)
# 显示结果
cv2.imshow('Image', result)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
相关推荐
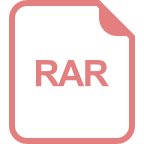
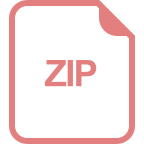
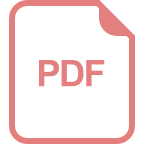
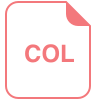
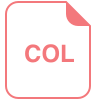
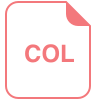
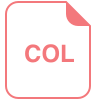
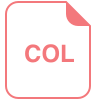









