pythonLSB加密算法代码
时间: 2024-05-13 14:12:48 浏览: 120
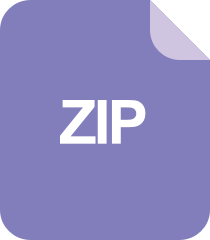
基于python的基于lsb算法与rsa算法的信息隐藏算法实现(django).zip
Python LSB加密算法是一种基于最低有效位隐写技术的加密算法。该算法可以将一张图片和一段文本进行加密,使得图片看起来并没有发生变化,但是文本已经被嵌入到了图片中。
以下是Python LSB加密算法的实现步骤:
1. 读取原始图片和需要加密的文本
2. 将文本转换成二进制字符串
3. 将二进制字符串嵌入到图片的最低有效位中
4. 保存加密后的图片
下面是Python实现代码:
```python
from PIL import Image
def encode_image(image_name, secret_message):
# Open image and convert to RGB format
img = Image.open(image_name).convert('RGB')
width, height = img.size
# Convert message to binary
secret_message = secret_message + "===" # Add stopping criteria
secret_message = ''.join([format(ord(i), "08b") for i in secret_message])
message_length = len(secret_message)
# Check if the message can be encoded in the image
if message_length > (width * height * 3) // 8:
raise ValueError("Error: Message too large to encode in image.")
# Encode the message in the image
encoded_img = img.copy()
index = 0
for row in range(height):
for col in range(width):
r, g, b = img.getpixel((col, row))
if index < message_length:
encoded_img.putpixel((col, row), (r - r % 2 + int(secret_message[index]), g - g % 2 + int(secret_message[index + 1]), b - b % 2 + int(secret_message[index + 2])))
index += 3
else:
break
if index >= message_length:
break
return encoded_img
def decode_image(image_name):
# Open image and get size
img = Image.open(image_name).convert('RGB')
width, height = img.size
# Decode the message from the image
binary_str = ""
for row in range(height):
for col in range(width):
r, g, b = img.getpixel((col, row))
binary_str += str(r % 2)
binary_str += str(g % 2)
binary_str += str(b % 2)
# Convert binary string to ASCII message
message = ""
for i in range(0, len(binary_str), 8):
byte = binary_str[i:i+8]
message += chr(int(byte, 2))
if message[-3:] == "===":
break
return message[:-3]
# Example usage
encoded_img = encode_image("original.png", "This is a secret message.")
encoded_img.save("encoded.png")
decoded_message = decode_image("encoded.png")
print(decoded_message)
```
阅读全文
相关推荐
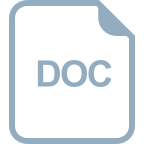
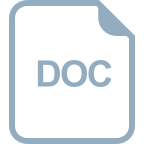
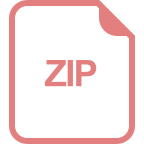
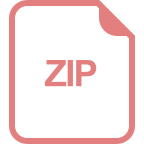
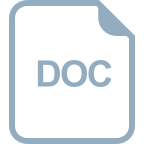
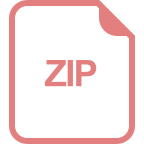
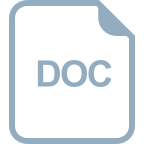
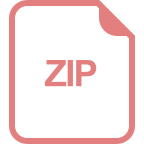
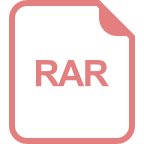
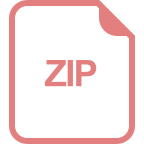
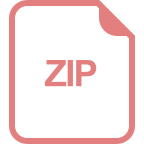
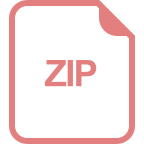
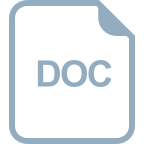
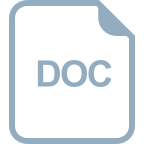
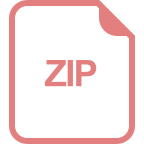
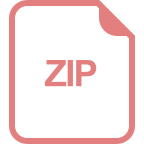
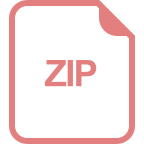
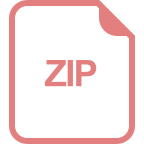