利用串的基本运算,编写一个算法删除串S1中所有的S2子串。 要求如下: 1、示例:S1=‘abcabc’,S2=‘ab’,执行后S1=‘cc’ 2、S2不能是空串 3、若S2不是S1的子串,需提示错误信息 用C++写出来
时间: 2024-10-10 13:13:12 浏览: 40
在C++中,我们可以使用双指针技巧来解决这个问题。这里有一个简单的算法:
```cpp
#include <string>
std::string removeSubstrings(std::string S1, std::string S2) {
if (S2.empty()) {
throw std::invalid_argument("Error: Substring S2 cannot be empty.");
}
int i = 0; // 指向S1的起始位置
while (i != S1.npos) {
// 查找S2是否出现在S1的剩余部分
size_t found = S1.find(S2, i);
if (found == std::string::npos) { // 如果找不到,直接返回S1
return S1;
}
// 删除找到的部分,并更新索引
S1.erase(i, found + S2.size());
i = found; // 从下一个字符开始查找
}
return S1;
}
// 示例
int main() {
std::string S1 = "abcabc";
std::string S2 = "ab";
try {
std::string result = removeSubstrings(S1, S2);
std::cout << "After removing " << S2 << ": " << result << std::endl;
} catch (const std::exception& e) {
std::cerr << e.what() << std::endl;
}
return 0;
}
```
这个函数首先检查S2是否为空,如果为空则抛出异常。然后它在一个while循环中查找S2在S1中的位置,每次找到了就删除该子串并移动搜索起点。如果S2不在S1中,则直接返回原始字符串。
注意,`std::string::npos`是一个特殊值,表示在字符串中找不到指定的子串。在C++中,`find`函数如果找不到子串会返回这个值。
阅读全文
相关推荐
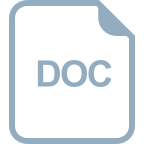
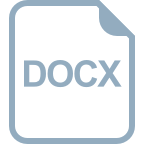
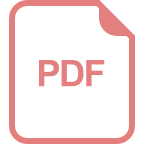















