用数据结构写个井字棋c语言
时间: 2024-01-14 17:03:37 浏览: 153
以下是使用C语言和数据结构实现井字棋的基本思路和步骤:
1. 定义棋盘数据结构
使用二维数组来表示棋盘,每个元素可以是空、X或O。
2. 初始化棋盘
在程序开始时,将棋盘上所有位置都设置为空。
3. 打印棋盘
使用循环遍历棋盘数组,根据每个元素的值打印出对应的符号。
4. 玩家下子
通过输入坐标来确定玩家下子的位置,将对应位置的元素设置为X。
5. 电脑下子
使用随机数生成器来确定电脑下子的位置,将对应位置的元素设置为O。
6. 判断胜负
在每次下子后,判断是否有一方已经连成三个相同的棋子。如果有,游戏结束,否则继续下一步。
7. 完成游戏
当游戏结束时,根据胜利方打印出对应的信息。
以下是实现井字棋的代码示例:
game.h文件:
```c
#ifndef GAME_H
#define GAME_H
#define BOARD_SIZE 3
typedef enum {EMPTY, X, O} Cell;
void init_board(Cell board[BOARD_SIZE][BOARD_SIZE]);
void print_board(Cell board[BOARD_SIZE][BOARD_SIZE]);
int player_move(Cell board[BOARD_SIZE][BOARD_SIZE]);
void computer_move(Cell board[BOARD_SIZE][BOARD_SIZE]);
int check_win(Cell board[BOARD_SIZE][BOARD_SIZE]);
#endif
```
game.c文件:
```c
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
#include "game.h"
void init_board(Cell board[BOARD_SIZE][BOARD_SIZE]) {
int i, j;
for (i = 0; i < BOARD_SIZE; i++) {
for (j = 0; j < BOARD_SIZE; j++) {
board[i][j] = EMPTY;
}
}
}
void print_board(Cell board[BOARD_SIZE][BOARD_SIZE]) {
int i, j;
for (i = 0; i < BOARD_SIZE; i++) {
for (j = 0; j < BOARD_SIZE; j++) {
switch (board[i][j]) {
case EMPTY:
printf(" ");
break;
case X:
printf("X");
break;
case O:
printf("O");
break;
}
if (j < BOARD_SIZE - 1) {
printf("|");
}
}
printf("\n");
if (i < BOARD_SIZE - 1) {
printf("-+-+-\n");
}
}
}
int player_move(Cell board[BOARD_SIZE][BOARD_SIZE]) {
int row, col;
printf("Enter row and column (1-3): ");
scanf("%d %d", &row, &col);
row--;
col--;
if (row < 0 || row >= BOARD_SIZE || col < 0 || col >= BOARD_SIZE) {
printf("Invalid move.\n");
return 0;
}
if (board[row][col] != EMPTY) {
printf("That square is already occupied.\n");
return 0;
}
board[row][col] = X;
return 1;
}
void computer_move(Cell board[BOARD_SIZE][BOARD_SIZE]) {
int row, col;
do {
row = rand() % BOARD_SIZE;
col = rand() % BOARD_SIZE;
} while (board[row][col] != EMPTY);
board[row][col] = O;
}
int check_win(Cell board[BOARD_SIZE][BOARD_SIZE]) {
int i, j;
for (i = 0; i < BOARD_SIZE; i++) {
if (board[i][0] != EMPTY && board[i][0] == board[i][1] && board[i][1] == board[i][2]) {
return board[i][0];
}
if (board[0][i] != EMPTY && board[0][i] == board[1][i] && board[1][i] == board[2][i]) {
return board[0][i];
}
}
if (board[0][0] != EMPTY && board[0][0] == board[1][1] && board[1][1] == board[2][2]) {
return board[0][0];
}
if (board[0][2] != EMPTY && board[0][2] == board[1][1] && board[1][1] == board[2][0]) {
return board[0][2];
}
return EMPTY;
}
```
test.c文件:
```c
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
#include "game.h"
int main() {
Cell board[BOARD_SIZE][BOARD_SIZE];
int turn = 0;
int winner = EMPTY;
srand(time(NULL));
init_board(board);
while (winner == EMPTY) {
printf("Turn %d:\n", turn + 1);
if (turn % 2 == 0) {
while (!player_move(board));
} else {
computer_move(board);
}
print_board(board);
winner = check_win(board);
turn++;
}
if (winner == X) {
printf("You win!\n");
} else if (winner == O) {
printf("Computer wins!\n");
} else {
printf("Tie game.\n");
}
return 0;
}
```
阅读全文
相关推荐
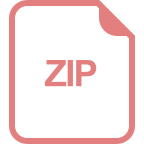
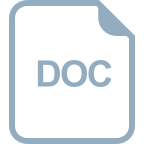
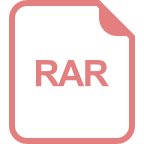
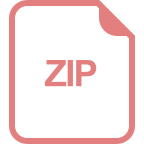
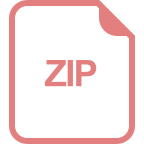
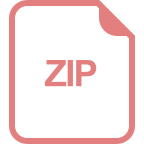
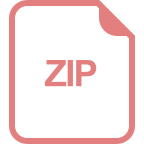
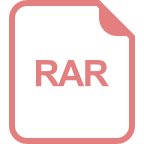
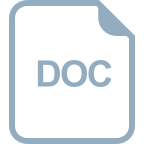
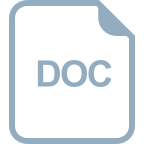
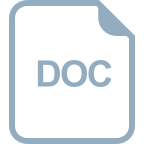
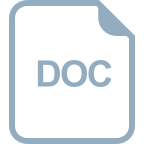
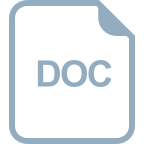
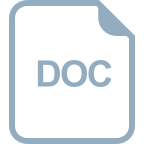
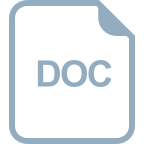

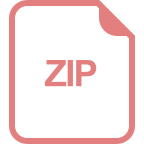