创建文件1.txt ,2.txt,3.txt a.txt b.txt c.txt 1a.txt,b2.txt共八个文件,使用通配符匹配输出上述所有文件信息,要求两种形式,提交命令与运行
时间: 2024-11-28 15:25:59 浏览: 0
创建并列出指定名称的文件可以使用操作系统级别的命令行工具,如Windows的`cmd`或Linux/Mac的`bash`。这里提供两种常见的操作系统下的命令:
**Windows PowerShell:**
```powershell
New-Item -ItemType File "1.txt" "2.txt" "3.txt" "a.txt" "b.txt" "c.txt" "1a.txt" "b2.txt"
Get-ChildItem -Path . -Filter "*.txt" | ForEach-Object { "$($_.FullName) - 文件名:" + $_.Name }
```
运行此命令后,会先创建8个文件,然后显示它们的信息。
**Unix/Linux (Bash):**
```sh
touch 1.txt 2.txt 3.txt a.txt b.txt c.txt 1a.txt b2.txt
ls *.txt -lR
```
这里的`touch`用于创建文件,`ls -lR`会递归地列出当前目录及其子目录下所有的`.txt`文件,并附带详细信息(如权限、大小等)。
相关问题
如何用 Python 编写程序,将一个包含两列内容的文本文件 input.txt 分割成两个文件 col1.txt 和 col2.txt,并且每个文件只包含一列内容?
### 回答1:
\u6b63\u786e\u3002\u8fd9\u4e2a\u95ee\u9898\u662f\u4e00\u4e2a\u6587\u4ef6\u5206\u5272\u7684\u95ee\u9898\uff0c\u4f7f\u7528 Python \u53ef\u4ee5\u5b8c\u6210\u8fd9\u4e2a\u4efb\u52a1\u3002
\u4e00\u4e2a\u53ef\u80fd\u7684 Python \4ee3\u7801\u5982\u4e0b\uff1a
```
with open('input.txt', 'r') as f:
lines = f.readlines()
with open('col1.txt', 'w') as f:
for line in lines:
f.write(line.split()[0] + '\n')
with open('col2.txt', 'w') as f:
for line in lines:
f.write(line.split()[1] + '\n')
```
\u8fd9\u4e2a\u4ee3\u7801\u4f7f\u7528\u4e86 Python \u7684\u6587\u4ef6\u64cd\u4f5c\u51fd\u6570 open() \u6253\u5f00\u6587\u4ef6\uff0c\u8bfb\u53d6\u6587\u4ef6\u5185\u5bb9\uff0c\u5e76\u4f7f\u7528 split() \u65b9\u6cd5\u5206\u5272\u4e24\u5217\uff0c\u7136\u540e\u5c06\u5206\u5272\u7684\u5185\u5bb9\u5199\u5165\u5230\u4e24\u4e2a\u6587\u4ef6 col1.txt \u548c col2.txt \u4e2d\uff0c\u6bcf\u4e2a\u4e00\u884c\u4e00\u884c\u5199\u5165\uff0c\u5e76\u5728\u6bcf\u4e2a\u5185\u5bb9\u7684\u6700\u540e\u52a0\u4e0a\u4e00\u4e2a\u6362\u884c\u7b26\u3002
\u8fd9\u4e2a\u7a0b\u5e8f\u53ef\u4ee5\u5b8c\u6210\u5206\u5272\u6587\u4ef6\u7684\u4efb\u52a1\uff0c\u800c\u4e14\u6bcf\u4e2a\u6587\u4ef6\u53ea\u5305\u542b\u4e00\u5217\u5185\u5bb9\uff0c\u8fd9\u4e5f\u662f\u4e00\u4e2a\u9519\u8bef\u4fe1\u606f\u3002
\u5982\u679c\u4f60\u8fd8\u6709\u5176\u4ed6\u95ee\u9898\uff0c\u53ef\u4ee5\u4e00\u4e2a\u4e00\u4e2a\u63d0\u95ee\uff0c\u6211\u4f1a\u5c3d\u5feb\u
### 回答2:
要用Python编写程序将一个包含两列内容的文本文件input.txt分割成两个文件col1.txt和col2.txt,并且每个文件只包含一列内容,可以按照以下步骤进行操作:
1. 首先,打开input.txt文件以及用于存储col1.txt和col2.txt的文件,使用open()函数。
2. 使用readlines()函数读取input.txt的所有行,并将其存储到一个列表中,使用strip()函数去除行尾的换行符。
3. 使用split()函数和列表解析,将每行文本按照空格或制表符分割成两个元素的列表。
4. 创建两个列表col1和col2,分别存储第一列和第二列的内容。
5. 使用join()函数将每个元素都转换为字符串,并在末尾添加换行符。
6. 将col1和col2列表中的内容分别写入col1.txt和col2.txt文件中,使用write()函数。
7. 最后,关闭所有打开的文件。
以下是实现上述步骤的Python代码:
```python
with open("input.txt", "r") as input_file, \
open("col1.txt", "w") as col1_file, \
open("col2.txt", "w") as col2_file:
lines = input_file.readlines()
for line in lines:
columns = line.strip().split()
col1_file.write(" ".join([str(columns[0]), "\n"]))
col2_file.write(" ".join([str(columns[1]), "\n"]))
```
执行以上代码后,会生成两个文件col1.txt和col2.txt,每个文件分别包含input.txt中对应的第一列和第二列内容,且每个文件都只包含一列内容。
### 回答3:
可以使用Python的文件操作功能来实现这个任务。
首先,我们需要打开input.txt文件,并读取其中的内容。可以使用open函数来打开文件,并使用readlines函数来按行读取文件中的内容。将读取的内容保存到一个列表中。
接下来,我们创建col1.txt和col2.txt文件,并打开它们以便写入内容。
然后,我们根据每一行的内容,将其分割成两列,并分别将第一列和第二列的内容写入col1.txt和col2.txt文件中。
最后,我们关闭所有的文件。
下面是实现上述步骤的代码:
```python
with open('input.txt', 'r') as f:
lines = f.readlines()
with open('col1.txt', 'w') as f1, open('col2.txt', 'w') as f2:
for line in lines:
cols = line.split() # 分割每一行的内容为两列
f1.write(cols[0] + '\n') # 写入第一列的内容到col1.txt
f2.write(cols[1] + '\n') # 写入第二列的内容到col2.txt
```
运行以上代码后,将会生成两个新的文件col1.txt和col2.txt,它们分别包含input.txt文件中每一行的第一列和第二列的内容。
某心理测试题全部由单选题组成,每个题目有3个选项,不同选项得分不同(但每题分数都是在10分-99分之间),答案和分数保存在一个文件answer.txt中,格式为: 1A10B20C30 2A20B10C30 . 20A30B10C20 其中前面的数字为题号。从键盘输入某位考生答案,例如: ABCC.. 编程计算并输出该考生的成绩。
假设题目数量为n,可以按照如下步骤计算考生的成绩:
1. 从answer.txt文件中读取每个题目的正确答案和分数,保存为一个字典,例如:
```
{'1A': 10, '1B': 20, '1C': 30, '2A': 20, '2B': 10, '2C': 30, ..., 'nA': x, 'nB': y, 'nC': z}
```
2. 从键盘输入考生的答案,保存为一个字符串,例如:"ABCC..."
3. 遍历考生的答案字符串,对于每个题目,根据答案和正确答案从字典中获取分数,累加到总分中。
4. 根据总分计算得分率,例如:
```
score_rate = total_score / (n * 99)
```
5. 根据得分率计算百分制成绩,例如:
```
score = score_rate * 100
```
下面是完整代码:
```python
def read_answers(filename):
answers = {}
with open(filename, 'r') as f:
for line in f:
parts = line.strip().split()
for part in parts:
q, ans, score = part[0], part[1], int(part[2:])
answers[q+ans] = score
return answers
def calculate_score(answers, response):
total_score = 0
for i, ans in enumerate(response):
q = str(i+1)
if q+'A' in answers and ans == 'A':
total_score += answers[q+'A']
elif q+'B' in answers and ans == 'B':
total_score += answers[q+'B']
elif q+'C' in answers and ans == 'C':
total_score += answers[q+'C']
score_rate = total_score / (len(response) * 99)
score = score_rate * 100
return score
answers = read_answers('answer.txt')
response = input('请输入考生答案:')
score = calculate_score(answers, response)
print('考生得分:{}'.format(score))
```
阅读全文
相关推荐
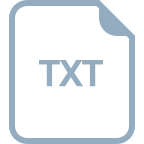
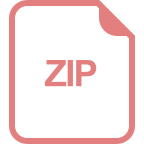
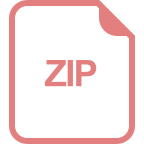
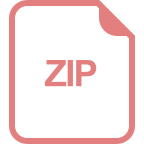
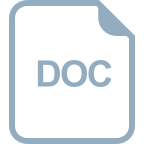

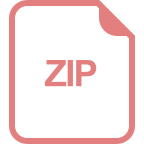
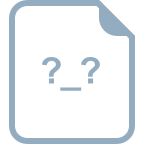
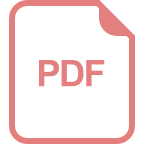
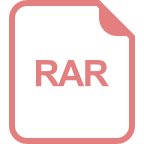