正则表达式计算字符串长度
时间: 2024-05-27 09:07:03 浏览: 163
正则表达式可以用来匹配字符串的模式,但是计算字符串长度不是正则表达式的功能。计算字符串长度可以直接使用编程语言提供的函数来实现,例如在Python中可以使用len()函数来计算字符串长度。如果您需要对字符串进行特定模式的匹配,可以使用正则表达式来实现。以下是一个Python示例,演示如何使用正则表达式来匹配特定模式的字符串:
```python
import re
string = "The quick brown fox jumps over the lazy dog"
pattern = r"[aeiou]"
matches = re.findall(pattern, string)
print(len(matches))
```
这段代码会输出字符串中所有元音字母的个数。其中,re.findall()函数使用正则表达式来匹配字符串中所有符合模式的子字符串,并将其以列表形式返回。最后,我们可以使用len()函数来计算列表长度,即为匹配到的子字符串个数。
相关问题
c语言,实现通过正则表达式查找字符串匹配的字符位置
在C语言中,你可以使用`pcre`库来实现正则表达式的匹配,并获取匹配的字符位置。这里有一个简单的示例,展示了如何使用`pcre`的`pcre_exec`函数以及其返回的信息来找到第一个匹配的位置:
```c
#include <stdio.h>
#include <string.h>
#include <pcre.h>
void find_match(const char* pattern, const char* input) {
PCRE *regex;
int erroffset = 0;
int rc;
int match_start, match_length;
regex = pcre_compile(pattern, 0, &erroffset, NULL, NULL);
if (regex == NULL) {
fprintf(stderr, "Error compiling pattern: %d-%s\n", erroffset, pcre_error_message());
return;
}
const char* startpos = input;
while ((rc = pcre_exec(regex, NULL, input, strlen(input), 0, 0, NULL, 0)) >= 0) {
match_start = rc;
match_length = rc - startpos; // 这里假设只有一个匹配,如果是多匹配,需要进一步解析ovec数组
printf("Match found at position: %d, length: %d\n", match_start, match_length);
input += match_length + 1; // 跳过已匹配部分,继续寻找下一个匹配
startpos = input; // 更新搜索起始位置
}
if (rc != PCRE_ERROR_NOMATCH) {
printf("No match found in the string.\n");
}
pcre_free(regex);
}
int main() {
const char* pattern = "[A-Za-z]+"; // 匹配连续的字母
const char* input = "Hello, World!";
find_match(pattern, input);
return 0;
}
```
在这个例子中,`find_match`函数接受一个正则表达式和一个输入字符串作为参数。`pcre_exec`会返回匹配开始的偏移量,我们从那里计算出匹配的长度。如果找不到匹配,`pcre_exec`将返回`PCRE_ERROR_NOMATCH`。
shell使用切片或正则表达式提取字符串中的任意位置和任意长度的数字
在shell脚本中,可以使用`cut`, `expr`或者更复杂的正则表达式库如`grep -oP`来提取特定位置和长度的数字。以下是一些常用的方法:
1. **`cut`命令**: 如果你知道数字在字符串的具体位置,你可以使用`cut`的字段选择语法。例如:
```bash
str="example1234"
num=$(echo $str | cut -d'-' -f2)
```
这里 `-d '-'` 指定分隔符为短横线,`-f2` 表示取第二个字段,也就是数字。
2. **`expr`和`=~`运算符**: 使用`=~`进行正则匹配,并结合`expr`计算匹配结果:
```bash
str="my_number_123"
num=$(expr substr "$str" 7 3)
```
`substr`用于截取从第7个字符开始,长度为3的子串,假设数字总是在某个固定的位数之后。
3. **正则表达式提取** (需要外部工具如`perl`):
```bash
perl -ne 'print if /^\d+/; print "$&\n"' <<< "$str"
```
`^\d+$` 匹配连续的数字,`$&` 取得整个匹配的数字。
阅读全文
相关推荐
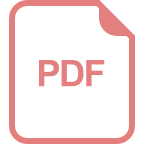
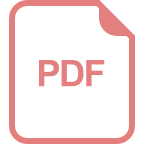
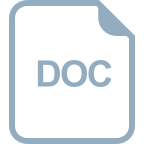
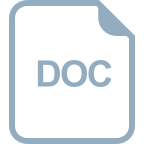
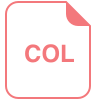
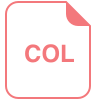
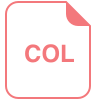
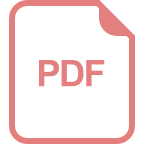
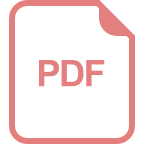
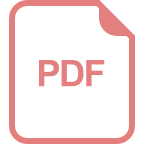
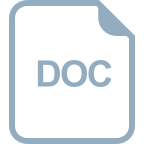
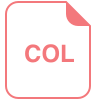
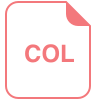