expected string or bytes-like object, got 'tuple'
时间: 2024-06-14 07:05:00 浏览: 326
出现"expected string or bytes-like object, got 'tuple'"的错误通常是因为代码中使用了一个期望是字符串或类似字节对象的函数,但实际上传递了一个元组。这个错误可能发生在使用正则表达式或字符串替换的过程中。
以下是两种可能导致这个错误的情况以及解决方法:
1. 使用正则表达式时出现错误:
```python
import re
pattern = r'\d+'
text = ('apple', 'banana', '123')
result = re.findall(pattern, text)
```
解决方法:在使用正则表达式时,需要将文本转换为字符串。可以使用`str()`函数将元组转换为字符串。
```python
result = re.findall(pattern, str(text))
```
2. 使用字符串替换时出现错误:
```python
text = ('apple', 'banana', '123')
new_text = text.replace('apple', 'orange')
```
解决方法:`replace()`函数只能用于字符串,不能用于元组。需要将元组转换为字符串后再进行替换。
```python
new_text = str(text).replace('apple', 'orange')
```
相关问题
报错expected string or bytes-like object, got float
这个错误通常是因为你使用了一个期望是字符串或字节的对象,但实际上传入了一个浮点数。可以检查一下你的代码,看看是否有地方将一个浮点数传给了期望字符串或字节的函数或方法。你可以尝试将浮点数转换为字符串或字节,然后再传给函数或方法。例如,可以使用 `str()` 或 `bytes()` 函数将浮点数转换为字符串或字节。
expected string or bytes-like object
这是一个错误提示,通常出现在代码中使用了需要字符串或字节对象作为输入参数,但实际给出的参数类型不符合要求的情况。你可以检查一下你的代码,看看是否有这样的错误发生了。如果有,需要针对具体情况进行相应的调整。
阅读全文
相关推荐
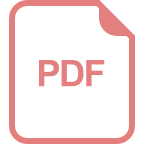
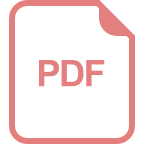











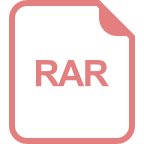
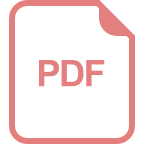
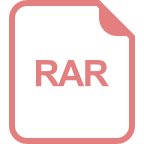