mybatis批量查询传入list
时间: 2023-08-18 16:12:24 浏览: 126
在 MyBatis 中进行批量查询时,可以传入一个 List 对象作为参数。以下是一个示例代码:
```java
public interface UserMapper {
List<User> getUserList(List<Integer> idList);
}
```
在 XML 映射文件中,可以使用 `<foreach>` 标签来处理这个 List 对象:
```xml
<select id="getUserList" resultType="User">
SELECT * FROM user
WHERE id IN
<foreach item="item" index="index" collection="list" open="(" separator="," close=")">
#{item}
</foreach>
</select>
```
在这个示例中,`getUserList` 方法接收一个 `List<Integer>` 参数 `idList`,然后在 SQL 查询中使用 `<foreach>` 标签将列表中的元素拼接成 IN 子句的条件。每个元素会被替换成 `#{item}`。
这样,你就可以通过传入一个 List 对象来进行批量查询了。
相关问题
mybatis批量查询传入list怎么写
你可以在mapper.xml文件中使用foreach标签来实现批量查询传入list的功能,具体的写法如下:
1. 在mapper接口中定义方法:
```
public List<User> getUsersByIdList(List<Integer> idList);
```
2. 在mapper.xml中实现方法:
```
<select id="getUsersByIdList" parameterType="java.util.List" resultMap="user">
select * from user where id in
<foreach item="item" index="index" collection="list" open="(" separator="," close=")">
#{item}
</foreach>
</select>
```
其中,parameterType指定参数类型为List,collection指定要遍历的集合,item和index分别为遍历时的当前元素和索引,open和close指定遍历的开头和结尾字符串,separator指定遍历时的分隔符。在查询参数中,可直接传入一个Integer类型的List。
希望以上回答能够帮到你。
mybatis修改数据传入list
MyBatis中,要传入List类型的参数进行批量修改操作,可以使用`<foreach>`标签来遍历List中的每个元素进行修改操作。
例如,假设有一个User对象,包含id、name和age三个属性,要批量修改User对象的name和age属性,可以按照以下步骤进行操作。
首先,在Mapper.xml文件中编写批量修改语句,例如:
```xml
<update id="updateBatch" parameterType="java.util.List">
update user set name=#{name}, age=#{age} where id=#{id}
</update>
```
其中,`parameterType="java.util.List"`表示传入的参数类型为List,每个元素都是要修改的对象。
然后,在Java代码中调用这个批量修改语句,例如:
```java
List<User> userList = new ArrayList<>();
// 添加要修改的用户对象到列表中
int result = sqlSession.update("updateBatch", userList);
```
其中,`sqlSession.update("updateBatch", userList)`表示执行批量修改操作,参数`updateBatch`为Mapper.xml中定义的id,`userList`为要修改的对象列表。
需要注意的是,在`<foreach>`标签中,可以使用`collection`属性指定要遍历的集合,`item`属性指定集合中的每个元素,`open`属性表示循环开始前的字符串,`close`属性表示循环结束后的字符串,`separator`属性表示每个元素之间的分隔符。例如:
```xml
<update id="updateBatch" parameterType="java.util.List">
update user
<set>
<foreach collection="list" item="user" separator=",">
name=#{user.name},
age=#{user.age}
</foreach>
</set>
where id=#{id}
</update>
```
其中,`collection="list"`表示遍历的集合为传入的List参数,`item="user"`表示集合中的每个元素为名为user的变量。在`<set>`标签中,使用`<foreach>`标签遍历集合中的每个元素,生成修改语句。`separator=","`表示每个元素之间用逗号分隔。
阅读全文
相关推荐
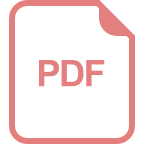

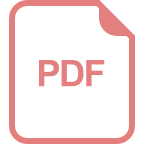









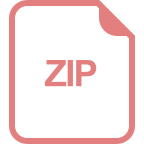