java保护人的隐私程序设计代码
时间: 2024-10-21 09:06:55 浏览: 40
在Java中设计保护个人隐私的程序,通常会涉及到数据加密、访问控制和匿名化处理等方面。这里提供一个简化的示例,展示如何使用`java.security`包下的`javax.crypto`库进行基本的加密操作:
```java
import javax.crypto.Cipher;
import javax.crypto.spec.SecretKeySpec;
import java.nio.charset.StandardCharsets;
import java.util.Base64;
public class PrivacyProtection {
private static final String ALGORITHM = "AES";
private static final String KEY = "your_secret_key"; // 使用实际的密钥
public static String encrypt(String plainText) throws Exception {
SecretKeySpec secretKey = new SecretKeySpec(KEY.getBytes(StandardCharsets.UTF_8), ALGORITHM);
Cipher cipher = Cipher.getInstance(ALGORITHM);
cipher.init(Cipher.ENCRYPT_MODE, secretKey);
byte[] encryptedBytes = cipher.doFinal(plainText.getBytes(StandardCharsets.UTF_8));
return Base64.getEncoder().encodeToString(encryptedBytes);
}
public static String decrypt(String encryptedText) throws Exception {
SecretKeySpec secretKey = new SecretKeySpec(KEY.getBytes(StandardCharsets.UTF_8), ALGORITHM);
Cipher cipher = Cipher.getInstance(ALGORITHM);
cipher.init(Cipher.DECRYPT_MODE, secretKey);
byte[] decryptedBytes = cipher.doFinal(Base64.getDecoder().decode(encryptedText));
return new String(decryptedBytes, StandardCharsets.UTF_8);
}
public static void main(String[] args) {
String originalMessage = "This is a sensitive message";
try {
String encrypted = encrypt(originalMessage);
System.out.println("Encrypted: " + encrypted);
String decrypted = decrypt(encrypted);
System.out.println("Decrypted: " + decrypted); // 输出: This is a sensitive message (假设解密成功)
} catch (Exception e) {
e.printStackTrace();
}
}
}
```
这个例子展示了如何使用对称加密(如AES)来加密和解密字符串,以保护用户的隐私信息。在实际应用中,你需要妥善保管和传输秘钥,并且在合适的地方实现访问权限控制。
阅读全文
相关推荐
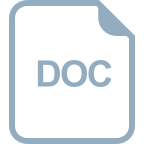
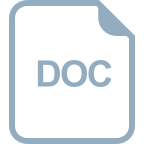
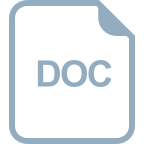


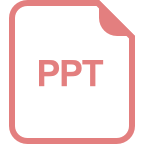
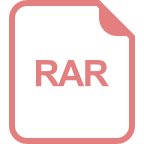
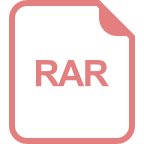
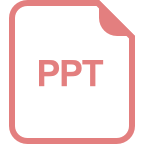
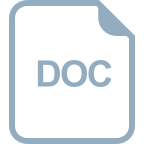
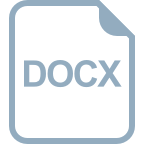
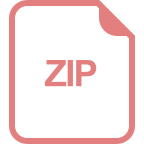
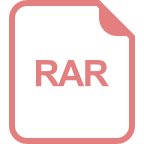
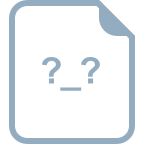
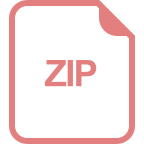
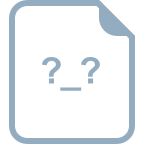
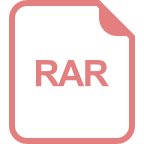
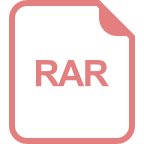
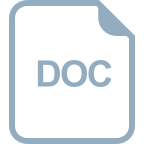