编写一个C程序,使用管道机制实现线程间的通信。
时间: 2024-10-21 12:13:06 浏览: 34
在C语言中,你可以使用`<unistd.h>`头文件中的`pipe()`、`fork()`和`close()`等函数来创建管道并进行父子进程之间的通信。以下是一个简单的例子,展示了如何在一个父进程中创建一个管道,然后在两个子进程中分别读写这个管道:
```c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <sys/types.h>
#define BUFFER_SIZE 100
int main() {
// 创建管道
int pipefd[2];
if (pipe(pipefd) == -1) {
perror("Failed to create pipe");
exit(EXIT_FAILURE);
}
pid_t child1_pid, child2_pid;
// 创建子进程1(writer)
if ((child1_pid = fork()) < 0) {
perror("Fork failed in parent process");
exit(EXIT_FAILURE);
}
if (child1_pid == 0) { // 子进程1
close(pipefd[1]); // 关闭写端
write(pipefd[0], "Hello from Child1", strlen("Hello from Child1") + 1); // 写入数据
printf("Child1 wrote to pipe and exiting.\n");
exit(0);
} else {
close(pipefd[0]); // 父进程关闭读端
// 创建子进程2(reader)
if ((child2_pid = fork()) < 0) {
perror("Fork failed in parent process");
exit(EXIT_FAILURE);
}
if (child2_pid == 0) { // 子进程2
char buffer[BUFFER_SIZE];
read(pipefd[1], buffer, BUFFER_SIZE); // 从管道读取数据
printf("Received message: %s\n", buffer);
printf("Child2 read from pipe and exiting.\n");
exit(0);
}
}
// 等待子进程结束
wait(NULL);
printf("Parent process completed.\n");
return 0;
}
```
在这个程序中,我们首先创建了一个管道(`pipefd[]`),然后创建了两个子进程。子进程1负责写入管道,而子进程2负责从管道读取。父进程则负责关闭不需要的那一端,并等待子进程完成。
阅读全文
相关推荐
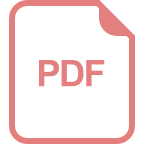
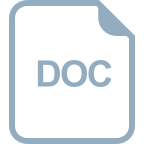
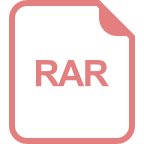
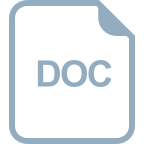
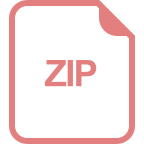
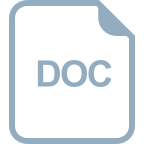
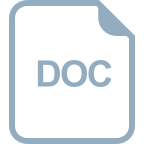
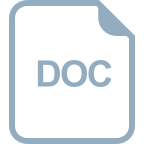
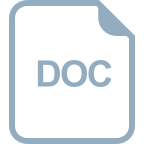
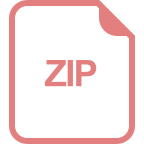
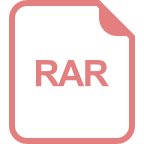
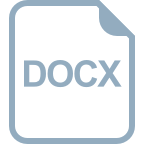
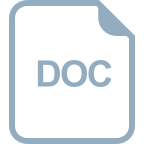
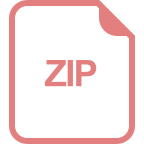
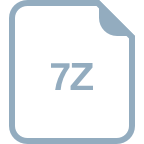
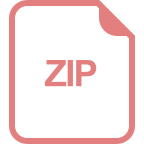
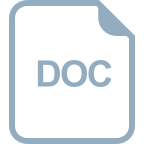
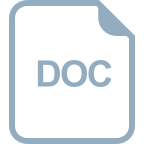