vue 随机生成100个名字,不重复, 例如 王x明形势展示,100个轮播每个3s随机获取
时间: 2024-11-06 07:35:57 浏览: 21
在Vue.js中,为了随机生成100个不重复的名字并实现轮播效果,你可以使用JavaScript的`Math.random()`函数结合数组和循环来生成名字,并配合Vue的生命周期钩子和`v-for`指令来实现轮播功能。这里是一个简单的示例:
首先,在数据中初始化一个空数组存储生成的名字:
```javascript
data() {
return {
names: [],
currentNameIndex: 0,
intervalId: null,
};
},
```
然后创建一个方法生成名字:
```javascript
methods: {
generateUniqueNames() {
const possibleChars = '王李赵陈吴';
for (let i = 0; i < 100; i++) {
let name = '';
while (name.length <= 2) { // 每个名字长度为2
const char = possibleChars[Math.floor(Math.random() * possibleChars.length)];
name += char;
}
if (!this.names.includes(name)) { // 确保名字不重复
this.names.push(name);
} else {
continue; // 如果重复则重试
}
}
},
},
```
在`mounted`生命周期钩子中,调用`generateUniqueNames`方法:
```javascript
mounted() {
this.generateUniqueNames();
},
```
最后,使用`v-for`来展示轮播效果:
```html
<div class="carousel">
<div v-for="(name, index) in names" :key="index">
<p>{{ `王${name}形势展示` }}</p>
</div>
</div>
<!-- 添加轮播定时器 -->
<script setup>
import { ref, onMounted } from 'vue';
const intervalRef = ref(null);
onMounted(() => {
this.intervalId = setInterval(() => {
if (this.currentNameIndex < this.names.length - 1) {
this.currentNameIndex++;
} else {
this.currentNameIndex = 0;
}
// 更新显示的名字
this.$forceUpdate();
}, 3000); // 设置轮播间隔为3秒
// 清除定时器时记得销毁应用
beforeDestroy(() => clearInterval(this.intervalId));
});
</script>
```
上面的代码会在页面加载完成后生成100个不重复的名字,并且在每次间隔3秒后切换到下一个名字。
阅读全文
相关推荐
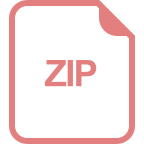
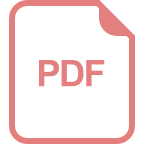
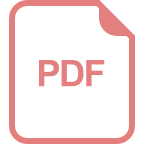

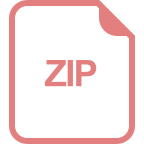
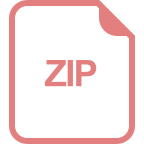
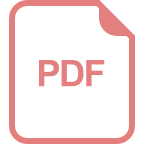
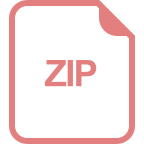
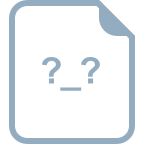
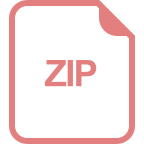
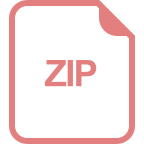
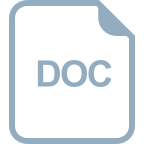


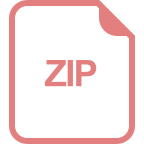