Java 获取本地文件真实格式
时间: 2024-11-15 20:22:59 浏览: 12
在Java中,获取本地文件的真实格式通常涉及到读取文件内容并解析其特定的元数据或结构。如果你需要确定文本文件、图像文件、PDF等的具体格式,你可以使用相应的库或者按照文件头信息来进行判断。
例如,对于文本文件,可以检查文件的开头字符,如`.txt`可能是纯文本,`.csv`可能是逗号分隔值,`.xml`可能是XML文档。
对于二进制文件,比如图片或音频,你可以利用一些库,如`java.awt.ImageIO`识别JPEG、PNG等图片格式,或者`javax.sound.sampled.AudioSystem`处理音频格式。
如果文件是特定格式的文件,如PDF、Word文档等,你可以使用专门针对这类文件格式解析的库,例如Apache POI用于Microsoft Office文档,iText库用于PDF。
这里有一个简单的示例,检测是否是JPEG图片:
```java
import java.io.File;
import javax.imageio.ImageIO;
public boolean isJpeg(File file) {
try {
ImageIO.read(file);
return true; // 文件可能是JPEG格式
} catch (IOException e) {
return false; // 解析失败,可能不是JPEG
}
}
```
不过,这只是一个基本的判断方法,更准确地识别文件格式通常需要依赖于特定库的API。
相关问题
java代码查找一个Azure的本地文件upload上传示例
以下是Java语言的Azure Blob Storage本地文件上传示例:
1. 首先需要安装Azure Blob Storage SDK。可以在Maven中添加以下依赖项:
```
<dependency>
<groupId>com.microsoft.azure</groupId>
<artifactId>azure-storage</artifactId>
<version>10.0.3</version>
</dependency>
```
2. 引用以下包:
```
import com.microsoft.azure.storage.CloudStorageAccount;
import com.microsoft.azure.storage.blob.CloudBlobClient;
import com.microsoft.azure.storage.blob.CloudBlobContainer;
import com.microsoft.azure.storage.blob.CloudBlockBlob;
```
3. 建立Blob服务连接:
```
String storageConnectionString = "DefaultEndpointsProtocol=https;AccountName=<your_account_name_here>;AccountKey=<your_account_key_here>;EndpointSuffix=core.windows.net";
CloudStorageAccount storageAccount = CloudStorageAccount.parse(storageConnectionString);
CloudBlobClient blobClient = storageAccount.createCloudBlobClient();
```
4. 获取容器引用:
```
CloudBlobContainer container = blobClient.getContainerReference("your_container_name_here");
```
5. 创建容器(如果不存在):
```
container.createIfNotExists();
```
6. 获取Blob引用:
```
CloudBlockBlob blob = container.getBlockBlobReference("your_blob_name_here");
```
7. 上传本地文件:
```
File file = new File("your_local_file_path_here");
blob.upload(new FileInputStream(file), file.length());
```
以上是一个基本的本地文件上传示例,你可以根据自己的需求进行修改和扩展。注意替换示例中的 `<your_account_name_here>`、`<your_account_key_here>`、`your_container_name_here`、`your_blob_name_here`、`your_local_file_path_here`为真实的值。
1.必答[简答题]编写一个Java类,实现将本地文件“province.txt"上传至“自己姓名目录”中,然后,编写方法实现将自己姓名目录中wordcount.txt文件的内容打印到控制台显示。
首先,我们需要创建一个简单的Java程序来完成这个任务。这个程序主要包括两个部分:文件上传和文件内容读取。
1. **文件上传**:
```java
import java.io.*;
public class FileUploader {
public static void uploadFile(String sourceFilePath, String destDirPath) {
try {
// 创建一个File对象表示源文件
File sourceFile = new File(sourceFilePath);
// 检查目标目录是否存在,不存在则创建
File destDir = new File(destDirPath);
if (!destDir.exists()) {
destDir.mkdir();
}
// 构建完整的文件路径并上传
String destFilePath = destDir.getAbsolutePath() + "/wordcount.txt";
Files.copy(sourceFile.toPath(), Paths.get(destFilePath));
System.out.println("文件 " + sourceFilePath + " 已经成功上传到 " + destFilePath);
} catch (IOException e) {
e.printStackTrace();
}
}
public static void main(String[] args) {
String username = "你的姓名"; // 替换为你的真实姓名
String sourceFilePath = "province.txt";
String destDirPath = username + "/"; // 用户名加斜杠作为目录名
uploadFile(sourceFilePath, destDirPath);
}
}
```
2. **文件内容读取**:
```java
public class FileReader {
public static void printContent(String filePath) {
try {
// 读取文件内容
File file = new File(filePath);
BufferedReader br = new BufferedReader(new FileReader(file));
String line;
while ((line = br.readLine()) != null) {
System.out.println(line);
}
br.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
为了在`main`方法中实现这两个功能,你需要将上述两段代码合并,并确保`province.txt`在运行时的类路径下可用。之后,你可以直接调用`uploadFile`方法上传文件,接着通过`printContent`方法读取并打印`wordcount.txt`的内容。
阅读全文
相关推荐
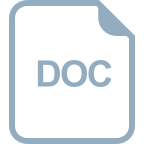
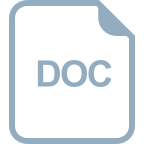
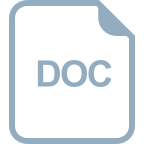
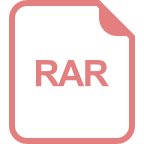
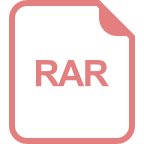
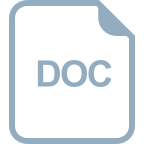
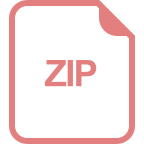
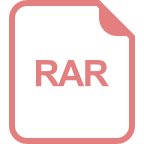
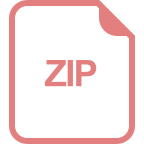
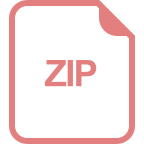
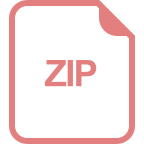
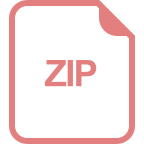
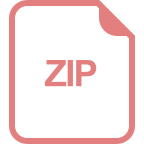
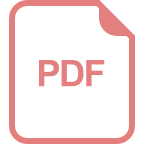
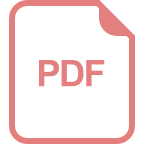
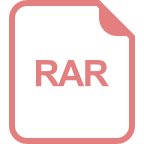
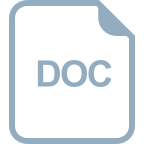