用python写一个字词拼写检查程序
时间: 2024-09-29 16:03:40 浏览: 52
在Python中编写一个简单的字词拼写检查程序,我们可以利用内置的`set`数据结构,结合`collections`模块中的`Counter`类。这个基本思路是检查输入单词是否只包含已知的字母,并且每个字母出现的次数与其在字典中的预期次数相匹配。
下面是一个简单的例子:
```python
import string
from collections import Counter
# 假设这是我们的英文词汇库
word_dict = set(' '.join(open('dictionary.txt', 'r').read().split()))
def spell_check(word):
# 移除标点并转换为小写
word = word.translate(str.maketrans('', '', string.punctuation)).lower()
# 使用Counter计算字符频率
counter_word = Counter(word)
# 如果所有字符频率都在字典中并且只有一次,那么就是正确的拼写
if all(counter_word[char] == 1 for char in counter_word) and word in word_dict:
return True
else:
return False
# 测试函数
test_words = ['correct', 'speling', 'misspelled']
for word in test_words:
print(f"{word}: {spell_check(word)}")
```
在这个程序中,`spell_check`函数接受一个单词作为输入,然后检查它是否在字典`word_dict`中,并且每个字符仅出现一次。如果满足这两个条件,就认为是正确的拼写。
阅读全文
相关推荐
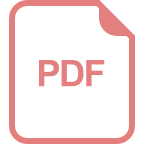
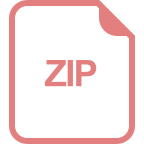
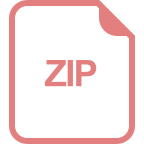









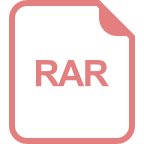
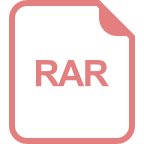
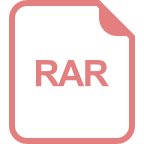
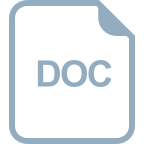
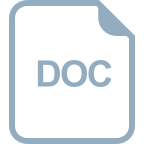

