typescript antdsidn 使用 checkbox组件使用indeterminate属性全选复写框代码
时间: 2024-09-28 13:13:05 浏览: 45
在 TypeScript 中,如果你想使用 Ant Design 的 Checkbox 组件实现一个可以切换全选状态的复写框,并支持 indeterminate 属性(表示选择状态不明),你可以这样做:
首先,你需要安装 Ant Design for TypeScript 和 @types/react,如果还没安装,可以使用 npm 或 yarn 进行安装:
```bash
npm install antd @types/react
# 或者
yarn add antd react ts @types/react
```
然后,在你的 TypeScript 文件中,创建一个自定义的 Checkbox 组件,添加 `indeterminate` 属性:
```typescript
import React from 'react';
import { Checkbox } from 'antd';
const CustomCheckbox: React.FC<{ indeterminate?: boolean }> = ({ indeterminate = false, ...props }) => {
const handleChange = (e: React.ChangeEvent<HTMLInputElement>) => {
if (!indeterminate) {
// 如果不是 indeterminate 状态,则切换全选/全不选
props.onChange(e.target.checked ? true : false);
}
};
return (
<Checkbox
{...props}
indeterminate={indeterminate}
onChange={handleChange}
/>
);
};
export default CustomCheckbox;
```
接下来,在你的应用中使用这个自定义的 Checkbox:
```tsx
import React from 'react';
import CustomCheckbox from './CustomCheckbox'; // 假设你在同一文件夹下
const MyComponent = () => {
const [allChecked, setAllChecked] = React.useState(false);
const handleCheckAll = () => {
setAllChecked(!allChecked);
};
return (
<>
{/* 列表或其他需要全选/全不选的 Checkbox */}
{items.map((item, index) => (
<CustomCheckbox
key={index}
checked={item.isChecked || allChecked} // item.isChecked 是每个项的状态
onChange={() => handleCheckAll()} // 当单个 Checkbox 变化时,更新所有项
indeterminate={allChecked && items.some(item => !item.isChecked)} // 根据已选项目判断是否为 indeterminate 状态
/>
))}
{/* 全选按钮 */}
<button onClick={handleCheckAll}>全选</button>
</>
);
};
```
阅读全文
相关推荐
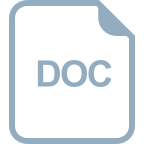
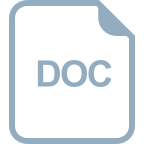
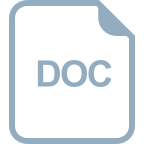




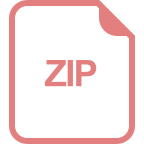
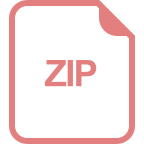
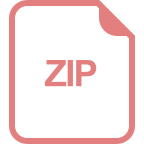
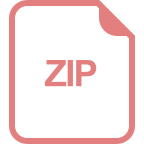
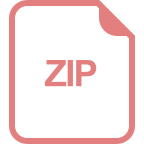
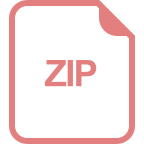
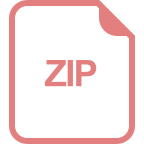
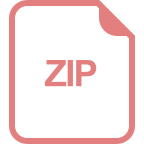
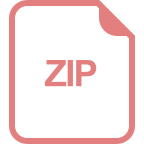
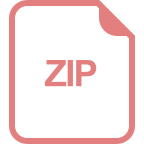
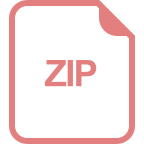