ByteArrayInputStream byteArrayInputStream = new ByteArrayInputStream(showVo.getOutputStream().toByteArray());这个方法很耗内存,有什么其他方法不耗内存,有没有详细的代码实现
时间: 2024-12-04 09:25:28 浏览: 9
`ByteArrayInputStream` 是 Java 中用于将字节数组转换为输入流的类。在您提供的代码片段中,`showVo.getOutputStream().toByteArray()` 返回一个字节数组,然后这个字节数组被传递给 `ByteArrayInputStream` 构造函数。这种方法确实会消耗内存,因为它需要将整个字节数组加载到内存中。
如果您希望避免这种内存消耗,可以考虑使用其他方法来处理数据流,例如直接从原始数据源读取数据,而不是先将数据全部加载到内存中。以下是一些可能的方法:
### 1. 使用 `PipedInputStream` 和 `PipedOutputStream`
这种方法通过管道连接两个流,从而避免了将整个数据加载到内存中。
```java
import java.io.*;
public class StreamExample {
public static void main(String[] args) throws IOException {
// 假设 showVo.getOutputStream() 返回的是 OutputStream
OutputStream outputStream = showVo.getOutputStream();
PipedInputStream pipedInputStream = new PipedInputStream();
PipedOutputStream pipedOutputStream = new PipedOutputStream(pipedInputStream);
// 启动一个新线程来写入数据
new Thread(() -> {
try (BufferedOutputStream bos = new BufferedOutputStream(pipedOutputStream)) {
outputStream.writeTo(bos); // 假设 writeTo 是一个自定义方法,将数据写入输出流
} catch (IOException e) {
e.printStackTrace();
}
}).start();
// 现在可以从 pipedInputStream 读取数据了
try (BufferedInputStream bis = new BufferedInputStream(pipedInputStream)) {
byte[] buffer = new byte[1024];
int bytesRead;
while ((bytesRead = bis.read(buffer)) != -1) {
// 处理读取的数据
}
}
}
}
```
### 2. 使用 `FileInputStream` 和临时文件
如果数据量较大,可以考虑将数据写入临时文件,然后从文件中读取。
```java
import java.io.*;
public class FileStreamExample {
public static void main(String[] args) throws IOException {
// 假设 showVo.getOutputStream() 返回的是 OutputStream
OutputStream outputStream = showVo.getOutputStream();
File tempFile = File.createTempFile("temp", ".tmp");
tempFile.deleteOnExit();
// 将数据写入临时文件
try (FileOutputStream fos = new FileOutputStream(tempFile)) {
outputStream.writeTo(fos); // 假设 writeTo 是一个自定义方法,将数据写入输出流
}
// 现在可以从临时文件中读取数据了
try (FileInputStream fis = new FileInputStream(tempFile)) {
byte[] buffer = new byte[1024];
int bytesRead;
while ((bytesRead = fis.read(buffer)) != -1) {
// 处理读取的数据
}
}
}
}
```
### 3. 使用 NIO 的 `Channels`
Java NIO(New I/O)提供了更高效的 I/O 操作方式,可以使用 `Channels` 来处理数据流。
```java
import java.io.*;
import java.nio.channels.*;
public class NIOExample {
public static void main(String[] args) throws IOException {
// 假设 showVo.getOutputStream() 返回的是 OutputStream
OutputStream outputStream = showVo.getOutputStream();
Pipe pipe = Pipe.open();
SinkChannel sinkChannel = pipe.sink();
SourceChannel sourceChannel = pipe.source();
// 启动一个新线程来写入数据
new Thread(() -> {
try (WritableByteChannel wbc = Channels.newChannel(outputStream)) {
sinkChannel.transferFrom(wbc, 0, Long.MAX_VALUE);
} catch (IOException e) {
e.printStackTrace();
} finally {
try {
sinkChannel.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}).start();
// 现在可以从 sourceChannel 读取数据了
try (ReadableByteChannel rbc = Channels.newChannel(new FileInputStream(sourceChannel))) {
ByteBuffer buffer = Byte
阅读全文
相关推荐
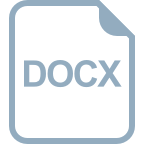
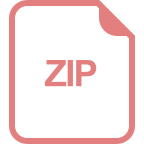
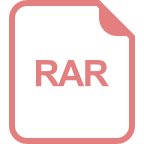
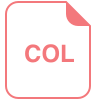
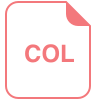
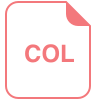







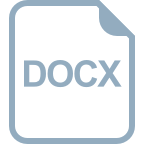
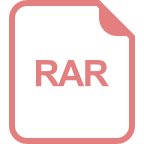