用栈判断回文C语言代码,将一半字符入栈
时间: 2024-10-10 10:05:47 浏览: 56
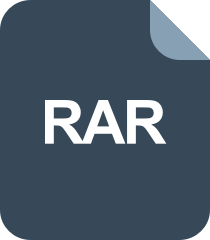
huiw.dsp.rar_回文栈和队列_用栈和队列编写判断回文的c语言程序
在 C 语言中,我们可以使用数组模拟栈的功能,下面是一个简单的示例,用于判断一个字符串是否是回文:
```c
#include <stdio.h>
#include <string.h>
#define MAX_STACK_SIZE 100
void push(char stack[], int top, char data) {
if (top == MAX_STACK_SIZE - 1) {
printf("Stack overflow\n");
return;
}
stack[top] = data;
top++;
}
char pop(char stack[], int top) {
if (top == -1) {
printf("Stack underflow\n");
return '\0';
}
char data = stack[top];
stack[top] = '\0'; // Mark the top as empty for next iteration
top--;
return data;
}
int isPalindrome(char str[]) {
int length = strlen(str);
int top = -1;
char stack[MAX_STACK_SIZE];
// Push half of the string into the stack
for (int i = 0; i < length / 2; i++) {
push(stack, top, str[i]);
}
// Compare the remaining characters with popped elements
for (int i = length / 2; i < length; i++) {
if (str[i] != pop(stack, top)) {
return 0; // Not a palindrome
}
}
return 1; // If all pairs match, it's a palindrome
}
int main() {
char str[] = "madam";
if (isPalindrome(str))
printf("%s is a palindrome.\n", str);
else
printf("%s is not a palindrome.\n", str);
return 0;
}
```
在这个例子中,我们首先计算字符串长度的一半并将前一半字符推入栈中。然后,我们在第二次循环中从字符串的后半部分开始,每次取出栈顶字符并与当前字符比较,如果它们不相等则立即返回 false。如果所有字符都能成功匹配,说明字符串是回文,返回 true。
阅读全文
相关推荐
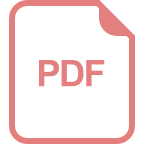
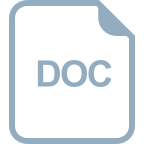















