用Python写一个FTP工具类,可以查看文件,上传,删除文件,创建目录,删除目录,其他需求你在补充
时间: 2024-04-25 13:24:16 浏览: 77
当然,我可以帮你编写一个简单的FTP工具类,用于查看文件、上传文件、删除文件、创建目录和删除目录。以下是一个示例代码:
```pythonimport ftplibclass FTPTool:
def __init__(self, host, username, password):
self.host = host self.username = username self.password = password self.ftp = ftplib.FTP()
def connect(self):
self.ftp.connect(self.host)
self.ftp.login(self.username, self.password)
def disconnect(self):
self.ftp.quit()
def list_files(self, directory='.'):
self.ftp.cwd(directory)
files = self.ftp.nlst()
return files def upload_file(self, local_file, remote_file):
with open(local_file, 'rb') as f:
self.ftp.storbinary(f'STOR {remote_file}', f)
def delete_file(self, remote_file):
self.ftp.delete(remote_file)
def create_directory(self, directory):
self.ftp.mkd(directory)
def delete_directory(self, directory):
self.ftp.rmd(directory)
```
使用示例:
```python# 创建FTPTool实例ftp_tool = FTPTool('your_ftp_host', 'your_username', 'your_password')
# 连接FTP服务器ftp_tool.connect()
# 查看文件列表files = ftp_tool.list_files()
print(files)
#上传文件ftp_tool.upload_file('local_file.txt', 'remote_file.txt')
# 删除文件ftp_tool.delete_file('remote_file.txt')
# 创建目录ftp_tool.create_directory('new_directory')
# 删除目录ftp_tool.delete_directory('new_directory')
# 断开连接ftp_tool.disconnect()
```
请注意,这只是一个简单的示例,具体的实现可能需要根据你的FTP服务器的要求进行调整。另外,为了确保安全性,请确保在实际使用时妥善处理密码等敏感信息。
阅读全文
相关推荐
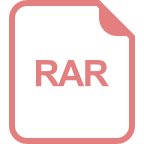
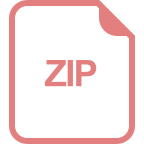
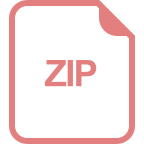
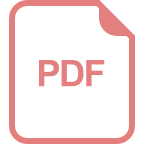
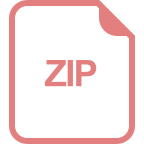
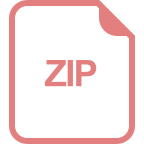
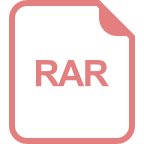
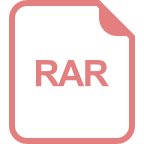
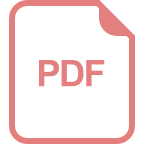
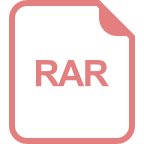
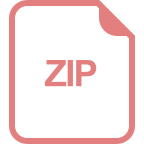
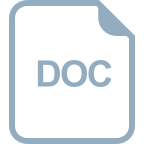
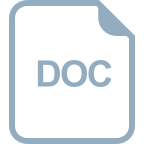
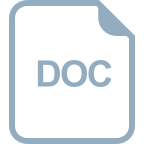
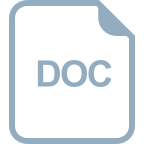
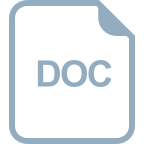
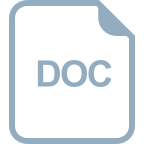
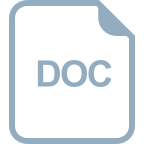