从键盘上依次输入21、18、30、75、42、56,逆序创建单链表(前插法),并输出单链表中的各元素值。按照上面题目,编写c语言代码
时间: 2024-10-06 13:04:07 浏览: 17
在C语言中,我们可以使用结构体定义链表节点,并结合前向递归(也称为迭代)的方式来创建一个逆序的单链表。下面是一个简单的例子,展示了如何实现这个功能:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义链表节点
struct ListNode {
int val; // 节点值
struct ListNode *next; // 指向下一個節點的指針
};
// 向链表末尾插入新节点
void insert(struct ListNode **head, int val) {
if (*head == NULL) {
*head = (struct ListNode*)malloc(sizeof(struct ListNode));
(*head)->val = val;
(*head)->next = NULL;
} else {
struct ListNode *newNode = (struct ListNode*)malloc(sizeof(struct ListNode));
newNode->val = val;
newNode->next = NULL;
struct ListNode *current = *head;
while (current->next != NULL) {
current = current->next;
}
current->next = newNode;
}
}
// 打印链表中的所有元素
void printList(struct ListNode *node) {
while (node != NULL) {
printf("%d ", node->val);
node = node->next;
}
printf("\n");
}
int main() {
struct ListNode *head = NULL;
// 输入数据并逆序插入链表
int input[6] = {21, 18, 30, 75, 42, 56};
for (int i = 5; i >= 0; i--) { // 从大到小输入
insert(&head, input[i]);
}
printf("逆序单链表中的各元素值: ");
printList(head);
return 0;
}
```
在这个代码里,我们首先定义了一个链表节点结构体 `ListNode`,包含一个整数值 `val` 和一个指向下一个节点的指针 `next`。`insert` 函数用于向链表的末尾添加新的节点,而 `printList` 函数则遍历整个链表并打印节点值。
在 `main` 函数中,我们创建一个空链表 `head`,然后将输入的数据从大到小逆序地逐个插入。最后,我们打印出链表的内容。
相关推荐
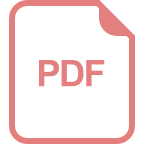
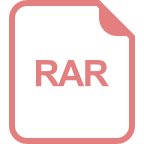
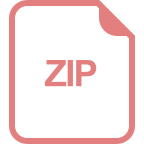















