如何实现两个结构体函数同时进行
时间: 2024-09-08 08:04:56 浏览: 82
在C语言中,同时让两个结构体函数并发执行通常是通过线程或者异步操作来实现的。这涉及到操作系统层面的知识和C库,例如POSIX线程(pthread)或者Windows API中的CreateThread函数。
以下是一个简单的示例,假设我们有两个结构体成员函数`func1()`和`func2()`:
```c
#include <pthread.h>
#include <stdio.h>
typedef struct {
void (*func1)();
void (*func2)();
} MyStruct;
void* func1_thread(void* arg) {
MyStruct* mystruct = (MyStruct*)arg;
mystruct->func1();
return NULL;
}
void* func2_thread(void* arg) {
MyStruct* mystruct = (MyStruct*)arg;
mystruct->func2();
return NULL;
}
// 定义结构体和函数指针
MyStruct myobj = { .func1 = &my_func1, .func2 = &my_func2 };
int main() {
pthread_t thread1, thread2; // 创建两个线程
// 启动线程
pthread_create(&thread1, NULL, func1_thread, &myobj);
pthread_create(&thread2, NULL, func2_thread, &myobj);
// 等待线程结束
pthread_join(thread1, NULL);
pthread_join(thread2, NULL);
printf("Both functions completed.\n");
return 0;
}
```
在这个例子中,`main`函数启动了两个新的线程,每个线程都对应一个结构体函数。`pthread_create`创建新线程,传入函数指针和结构体指针作为参数。
注意:为了保证数据的一致性和避免竞态条件,你可能还需要对共享的结构体变量加上互斥锁 (`pthread_mutex_t`) 或其他同步机制。
阅读全文
相关推荐
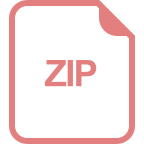
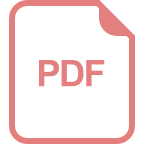
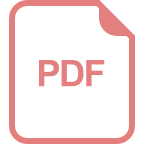
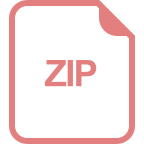
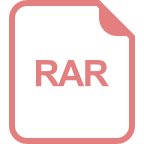
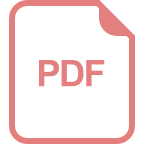
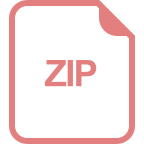
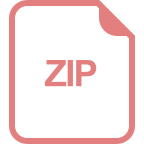
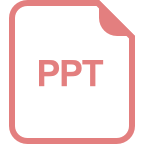
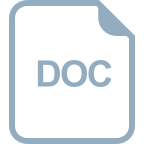
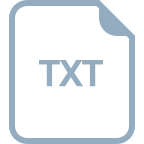
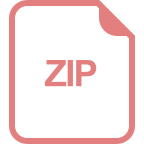
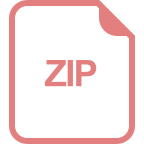
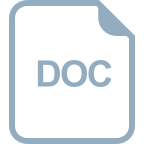
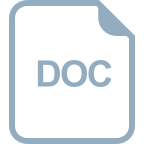
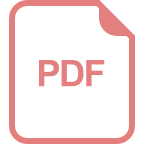
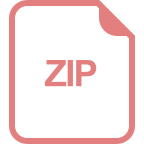